16
Graphics / SOLVED - [Thor] - Strange Error works then doesn't work no code change?
« on: July 16, 2012, 08:47:12 am »
I am getting a crash in my game and the console is getting this message printed in it..
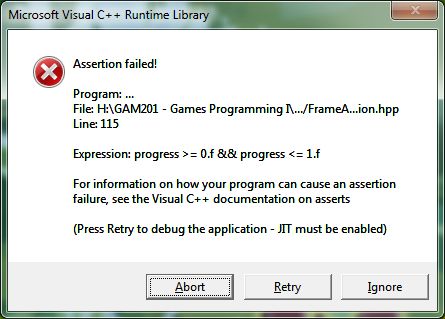
The strange thing is the crash only happens sometimes. If I compile, it will run, and then I run it a few times and suddenly it will crash with this error, with no code change at all. If I compile a release version, same thing happens, I can just sit there running the exe, and 1/2 the time it works.. the other 1/2 it crashes.
Anyideas?
Here is my code if it helps.
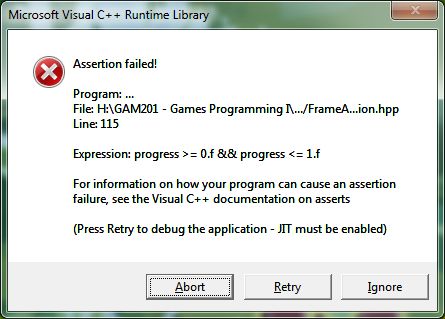
Code: [Select]
Assertion failed: progress >= 0.f && progress <= 1.f, file h:\nom!\_xternlibs\sfml2\include\thor\animation\frameanimation.hpp, line 115
The strange thing is the crash only happens sometimes. If I compile, it will run, and then I run it a few times and suddenly it will crash with this error, with no code change at all. If I compile a release version, same thing happens, I can just sit there running the exe, and 1/2 the time it works.. the other 1/2 it crashes.
Anyideas?
Here is my code if it helps.
#include "goPac.h"
goPac::goPac()
: _nextDirection(STOPPED)
, _velocity(10.0f)
, _maxVelocity(100.0f)
{
_PACsprite.setTexture(*TextureManager::getInstance().rSpriteSheet());
thor::FrameAnimation defaultAnim;
defaultAnim.addFrame(1.f, sf::IntRect(40, 80, 40, 40));
// Create animation: CHOMP
thor::FrameAnimation chomp;
chomp.addFrame(1.f, sf::IntRect(120, 80, 40, 40));
chomp.addFrame(1.5f, sf::IntRect(160, 80, 40, 40));
chomp.addFrame(1.f, sf::IntRect(120, 80, 40, 40));
chomp.addFrame(2.f, sf::IntRect(40, 80, 40, 40));
animPac.setDefaultAnimation(defaultAnim, sf::seconds(1.f));
animPac.addAnimation("chomp", chomp, sf::seconds(0.4f));
}
goPac::~goPac()
{
}
void goPac::Update(sf::RenderWindow& rw, sf::Event& _event, float TimeElapsed)
{
rw.pollEvent(_event);
rw.setKeyRepeatEnabled(false);
if (_event.type == sf::Event::KeyPressed)
{
if (_event.key.code == sf::Keyboard::Up || _event.key.code == sf::Keyboard::W || _event.key.code == sf::Keyboard::Num8)
{
debug("UP");
playAnimation();
}
if (_event.key.code == sf::Keyboard::Left || _event.key.code == sf::Keyboard::A || _event.key.code == sf::Keyboard::Num4)
{
debug("LEFT");
}
if (_event.key.code == sf::Keyboard::Right || _event.key.code == sf::Keyboard::D || _event.key.code == sf::Keyboard::Num6)
{
debug("RIGHT");
}
if (_event.key.code == sf::Keyboard::Down || _event.key.code == sf::Keyboard::S || _event.key.code == sf::Keyboard::Num2)
{
debug("DOWN");
}
}
animPac.update(animClock.restart());
animPac.animate(_PACsprite);
}
void goPac::playAnimation()
{
debug("playAnimation();");
animPac.update(animClock.restart());
animPac.playAnimation("chomp", true);
}
void goPac::Draw(sf::RenderWindow& rw)
{
rw.draw(_PACsprite);
}
goPac::goPac()
: _nextDirection(STOPPED)
, _velocity(10.0f)
, _maxVelocity(100.0f)
{
_PACsprite.setTexture(*TextureManager::getInstance().rSpriteSheet());
thor::FrameAnimation defaultAnim;
defaultAnim.addFrame(1.f, sf::IntRect(40, 80, 40, 40));
// Create animation: CHOMP
thor::FrameAnimation chomp;
chomp.addFrame(1.f, sf::IntRect(120, 80, 40, 40));
chomp.addFrame(1.5f, sf::IntRect(160, 80, 40, 40));
chomp.addFrame(1.f, sf::IntRect(120, 80, 40, 40));
chomp.addFrame(2.f, sf::IntRect(40, 80, 40, 40));
animPac.setDefaultAnimation(defaultAnim, sf::seconds(1.f));
animPac.addAnimation("chomp", chomp, sf::seconds(0.4f));
}
goPac::~goPac()
{
}
void goPac::Update(sf::RenderWindow& rw, sf::Event& _event, float TimeElapsed)
{
rw.pollEvent(_event);
rw.setKeyRepeatEnabled(false);
if (_event.type == sf::Event::KeyPressed)
{
if (_event.key.code == sf::Keyboard::Up || _event.key.code == sf::Keyboard::W || _event.key.code == sf::Keyboard::Num8)
{
debug("UP");
playAnimation();
}
if (_event.key.code == sf::Keyboard::Left || _event.key.code == sf::Keyboard::A || _event.key.code == sf::Keyboard::Num4)
{
debug("LEFT");
}
if (_event.key.code == sf::Keyboard::Right || _event.key.code == sf::Keyboard::D || _event.key.code == sf::Keyboard::Num6)
{
debug("RIGHT");
}
if (_event.key.code == sf::Keyboard::Down || _event.key.code == sf::Keyboard::S || _event.key.code == sf::Keyboard::Num2)
{
debug("DOWN");
}
}
animPac.update(animClock.restart());
animPac.animate(_PACsprite);
}
void goPac::playAnimation()
{
debug("playAnimation();");
animPac.update(animClock.restart());
animPac.playAnimation("chomp", true);
}
void goPac::Draw(sf::RenderWindow& rw)
{
rw.draw(_PACsprite);
}