Today I've been trying to implement a in-game console system for my game, I was just giving it a rectangle background, but when I ran the game it crashes as soon as I pressed F12 to open the console, and gave me ther error :
UntitledCppGame.exe has triggered a breakpoint.
When pressing the "break" button I was greeted by this page in visual studio :
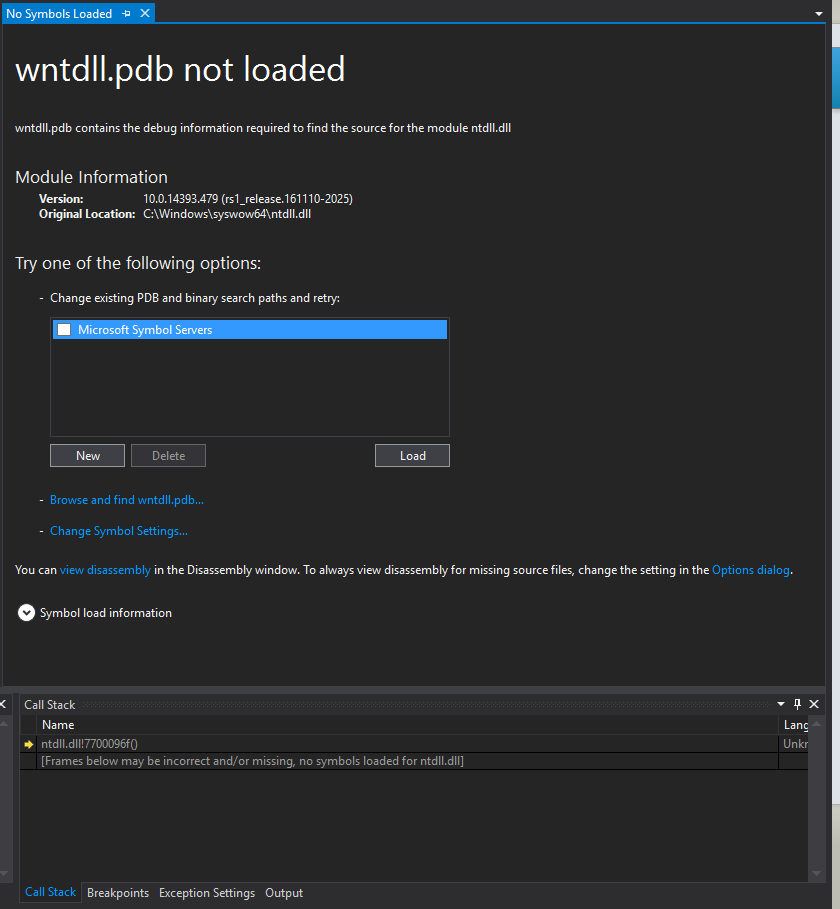
And that is all information that Visual Studio gives me about the crash, and I have no idea at all how to find out what's causing it.
Here's the head and cpp file for the Console system :
Header : #pragma once
#include "Input.h"
#include "BoxSprite.h"
class Console
{
public:
Console();
~Console();
void Initialize();
void OpenConsole();
void CloseConsole();
void Draw();
void Update();
void HandleEvents();
bool IsConsoleOpen();
void PrintMessage(std::string file, std::string msg);
void PrintWarning(std::string file, std::string msg);
void PrintError(std::string file, std::string msg);
void PrintFatal(std::string file, std::string msg);
private:
const int M_CONSOLE_OPEN_BIND = sf::Keyboard::F12;
bool m_isConsoleOpen;
bool m_isConsoleReady = false;
BoxSprite m_consoleRect;
};
Source : #include "Console.h"
#include "Globals.h"
Console gConsole;
Console::Console()
{
}
Console::~Console()
{
}
void Console::Initialize()
{
m_consoleRect = BoxSprite(Vector2(gGraphics.GetWindow().getSize().x, gGraphics.GetWindow().getSize().y / 3), Vector2(0, 0), Color::White);
m_isConsoleReady = true;
}
void Console::Draw()
{
if (m_isConsoleOpen == true)
{
m_consoleRect.Draw();
}
}
void Console::Update()
{
}
void Console::HandleEvents()
{
if (gInput.isKeyDown(M_CONSOLE_OPEN_BIND))
{
if (IsConsoleOpen() == true)
{
CloseConsole();
}
else
{
OpenConsole();
}
}
}
bool Console::IsConsoleOpen()
{
return m_isConsoleOpen;
}
void Console::OpenConsole()
{
}
void Console::CloseConsole()
{
}
void Console::PrintMessage(std::string file, std::string msg)
{
}
void Console::PrintWarning(std::string file, std::string msg)
{
}
void Console::PrintError(std::string file, std::string msg)
{
}
void Console::PrintFatal(std::string file, std::string msg)
{
}