1
This section allows you to view all posts made by this member. Note that you can only see posts made in areas you currently have access to.
Pages: [1] 2
2
Graphics / time variation when loading textures of different sizes?
« on: April 03, 2022, 01:38:21 pm »
hello! why does loading a big texture (16384x16384) takes so much more time than loading 16 textures of 4096x4096 (if the sum of the texture sizes is the same in the end)?
in this code:
in my computer (not a high-end) it took more than two minutes to load the big texture, and about 15 seconds to load all the small ones. I suspect it could be something related to the video board needing a contiguous block of memory, but I'm not sure.
anyway, can we somehow take advantage of that to make loading times faster? i tried drawing all the small ones in a RenderTexture, but loading it to a sf::Texture ended up taking much more time (about 5min)
in this code:
#include <SFML/Graphics.hpp>
#include <iostream>
int main(){
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML");
sf::Clock clock;
sf::Image big_img, small_img;
sf::Texture big_tex, small_tex[16];
big_img.create(16384, 16384);
small_img.create(4096, 4096);
clock.restart();
big_tex.loadFromImage(big_img);
std::cout << "big_tex loading time: " << clock.restart().asMilliseconds() << " ms" << std::endl;
for (int n=0; n<16; n++){
small_tex[n].loadFromImage(small_img);
std::cout << "small_tex[" << n << "] loading time: " << clock.restart().asMilliseconds() << " ms" << std::endl;
}
return 0;
}
#include <iostream>
int main(){
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML");
sf::Clock clock;
sf::Image big_img, small_img;
sf::Texture big_tex, small_tex[16];
big_img.create(16384, 16384);
small_img.create(4096, 4096);
clock.restart();
big_tex.loadFromImage(big_img);
std::cout << "big_tex loading time: " << clock.restart().asMilliseconds() << " ms" << std::endl;
for (int n=0; n<16; n++){
small_tex[n].loadFromImage(small_img);
std::cout << "small_tex[" << n << "] loading time: " << clock.restart().asMilliseconds() << " ms" << std::endl;
}
return 0;
}
in my computer (not a high-end) it took more than two minutes to load the big texture, and about 15 seconds to load all the small ones. I suspect it could be something related to the video board needing a contiguous block of memory, but I'm not sure.
anyway, can we somehow take advantage of that to make loading times faster? i tried drawing all the small ones in a RenderTexture, but loading it to a sf::Texture ended up taking much more time (about 5min)

3
SFML website / a "beginners" board in forum?
« on: December 17, 2020, 10:40:40 pm »
hi
I'd like to know what other members think of having a beginners' board in the 'Help' forum. i've seen lately some questions related mostly to C++, but still pertinet to SFML. which is nice, because it shows that some people are really trying to get the hang of programming, instead of just going for ready game engines like Unity.
so please, opinions.
I'd like to know what other members think of having a beginners' board in the 'Help' forum. i've seen lately some questions related mostly to C++, but still pertinet to SFML. which is nice, because it shows that some people are really trying to get the hang of programming, instead of just going for ready game engines like Unity.
- there are so many experienced developers here, and it would be a shame if thet didn't share their knowledge with the new programmers. unfortunately, it seems that not having such board discourages beginner questions, since even 'General' board expects you to have a good knowledge of C++, and then ask only SFML questions.
- altough there are specific forums for plain C++ with beginners' sections, you can't expect people there to know SFML. however, we can expect people in SFML forums to know C++.
- I know that some people would say "first you learn C++, and only then SFML". that's partially right. you can't use SFML if you don't know how to operate a variable. but why not learn both at the same time after the basics? people want to learn how they can make their games, not how to make boring command-line programs that simulate airplanes landing and taking off.
- the questions, as I said, are being made anyway. and will continue to, we having a beginners board or not.
so please, opinions.
4
General / drawing derived classes
« on: August 30, 2020, 05:15:31 pm »
hi
i believe this is a newbie issue related to SFML structure and C++
if I have a class derived from two drawables, like this:
how can I draw this class using a sf::RenderWindow? if I try, for example
thanks!
i believe this is a newbie issue related to SFML structure and C++
if I have a class derived from two drawables, like this:
class Board : public sf::Text, public sf::Sprite{
///etc;
};
///etc;
};
how can I draw this class using a sf::RenderWindow? if I try, for example
window.draw(board.Sprite);
I get an error saying invalid use of ‘sf::Sprite::Sprite’
but how do I make the window draw the sprite and then the text? is it mandatory that i override the draw function in Board class, pass a window reference, and then draw each one of the drawables from inside the class?thanks!
5
General / [SOLVED] need a better collision idea
« on: July 11, 2020, 04:54:41 pm »
hello!
I'm creating this city like game, but having some trouble with collision between the cursor and the building tiles.
my first idea (which is working now) was to detect collision with only the ground area of each building. but its extremely counter intuitive. video explaining:
by 7 seconds I move the cursor over a building, but the selected tile is the ground behind it.
I need some insight on how to make a better collision detection, considering that buildings have different heights (and street tiles have almost no height at all).
observation: the tiles are made of sf::Quads (shaped as parallelograms/diamonds, NOT rectangles) in a sf::VertexArray.
original tile image:
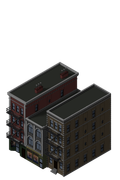
tiles boundings before rotating the view (notice that most buildings won't use all the tile size).
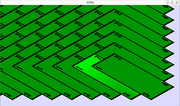
I don't know if it is of any help, but the building tiles before rotating the view:
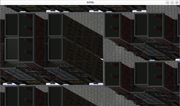
and after rotating:
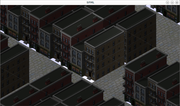
grid lines are for reference.
any idea is apreciated. thanks in advance!
I'm creating this city like game, but having some trouble with collision between the cursor and the building tiles.
my first idea (which is working now) was to detect collision with only the ground area of each building. but its extremely counter intuitive. video explaining:
by 7 seconds I move the cursor over a building, but the selected tile is the ground behind it.
I need some insight on how to make a better collision detection, considering that buildings have different heights (and street tiles have almost no height at all).
observation: the tiles are made of sf::Quads (shaped as parallelograms/diamonds, NOT rectangles) in a sf::VertexArray.
original tile image:
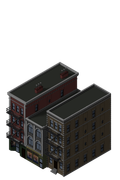
tiles boundings before rotating the view (notice that most buildings won't use all the tile size).
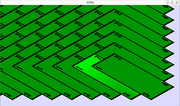
I don't know if it is of any help, but the building tiles before rotating the view:
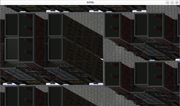
and after rotating:
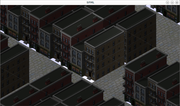
grid lines are for reference.
any idea is apreciated. thanks in advance!

6
Graphics / strange behaviour with View and Viewport
« on: October 25, 2019, 02:52:03 pm »
hello
I'm having trouble understanding this Views and Viewport situation:
in this code, the text appears while the mouse cursor is inside view_one, which occupies 100% of the height and 80% of the width (because the Viewport is (0, 0, 0,8, 1)):
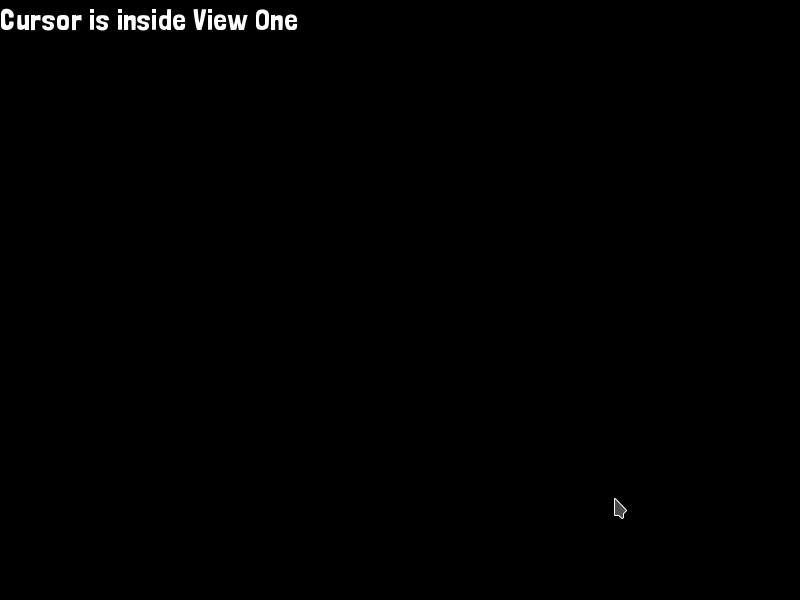
it works. but if I resize the view_one,
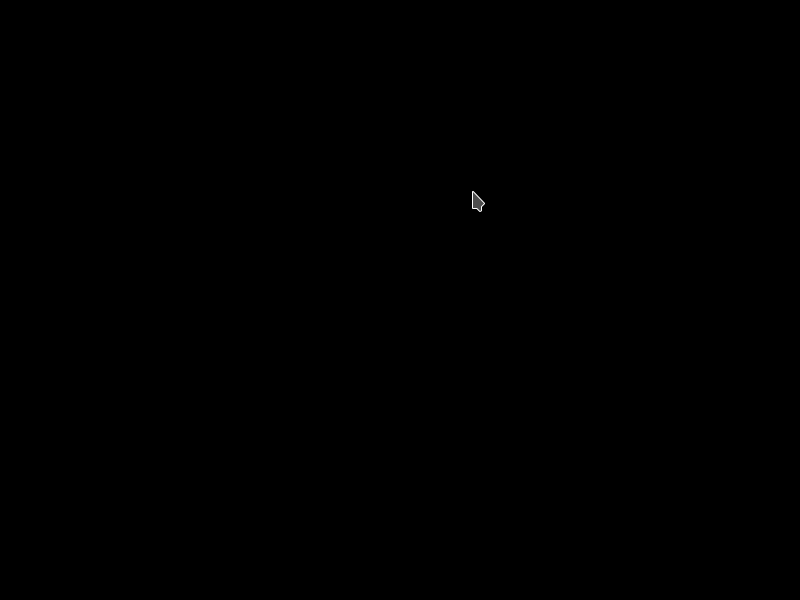
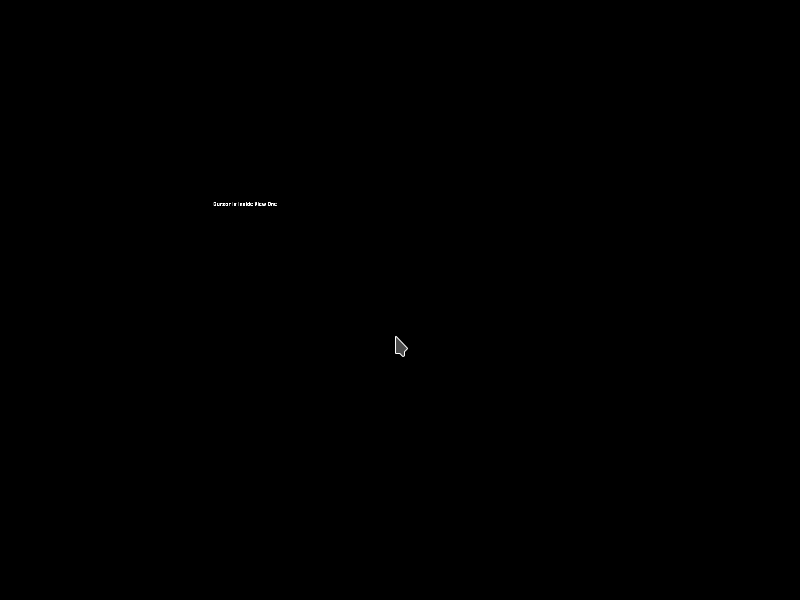
is that supposed to happen? how do I keep the correct proportions, so I can always check if the cursor is inside a given sf::View?
EDIT: I know that the VIEW should be resized, and with it the text inside. but the Viewport should not, I think. notice how the viewport shrinks to almost half the window size in the second image
EDIT 2: it works if I use
I'm having trouble understanding this Views and Viewport situation:
in this code, the text appears while the mouse cursor is inside view_one, which occupies 100% of the height and 80% of the width (because the Viewport is (0, 0, 0,8, 1)):
#include <SFML/Graphics.hpp>
int main(){
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Views");
sf::View view_one, view_two;
view_one.setViewport(sf::FloatRect(0, 0, 0.8, 1));
view_two.setViewport(sf::FloatRect(0.8, 0, 0.2, 1));
sf::Font font;
font.loadFromFile("LondrinaSolid-Regular.otf");
sf::Text text("Cursor is inside View One", font);
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if(event.type == sf::Event::Closed) window.close();
}
sf::Vector2f pos_in_view = window.mapPixelToCoords(sf::Mouse::getPosition(window), view_one);
window.clear();
if (sf::IntRect(view_one.getCenter().x-view_one.getSize().x/2, view_one.getCenter().y-view_one.getSize().y/2, view_one.getCenter().x+view_one.getSize().x/2, view_one.getCenter().y+view_one.getSize().y/2).contains(sf::Vector2i(pos_in_view))){
window.setView(view_one);
window.draw(text);
}
window.display();
}
return 0;
}
int main(){
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Views");
sf::View view_one, view_two;
view_one.setViewport(sf::FloatRect(0, 0, 0.8, 1));
view_two.setViewport(sf::FloatRect(0.8, 0, 0.2, 1));
sf::Font font;
font.loadFromFile("LondrinaSolid-Regular.otf");
sf::Text text("Cursor is inside View One", font);
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if(event.type == sf::Event::Closed) window.close();
}
sf::Vector2f pos_in_view = window.mapPixelToCoords(sf::Mouse::getPosition(window), view_one);
window.clear();
if (sf::IntRect(view_one.getCenter().x-view_one.getSize().x/2, view_one.getCenter().y-view_one.getSize().y/2, view_one.getCenter().x+view_one.getSize().x/2, view_one.getCenter().y+view_one.getSize().y/2).contains(sf::Vector2i(pos_in_view))){
window.setView(view_one);
window.draw(text);
}
window.display();
}
return 0;
}
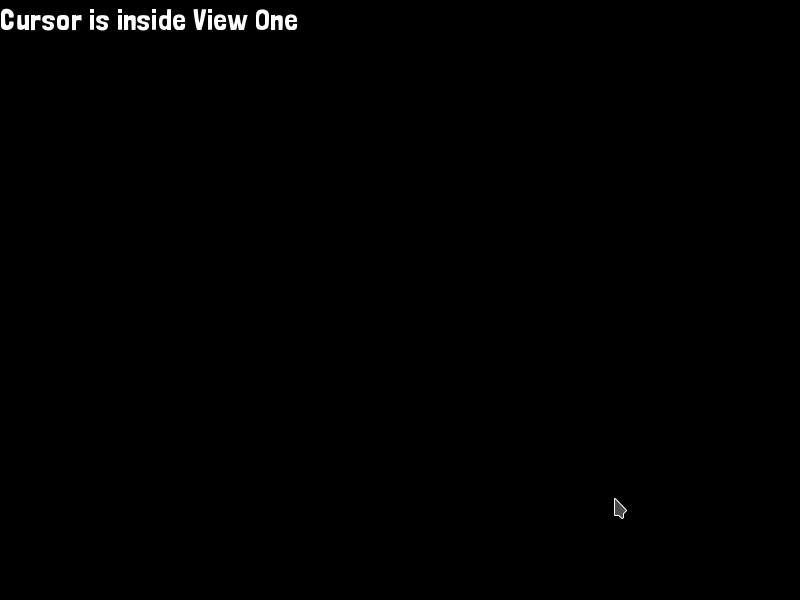
it works. but if I resize the view_one,
view_one.setSize(3000, 3000);
the viewport seems to be resized too 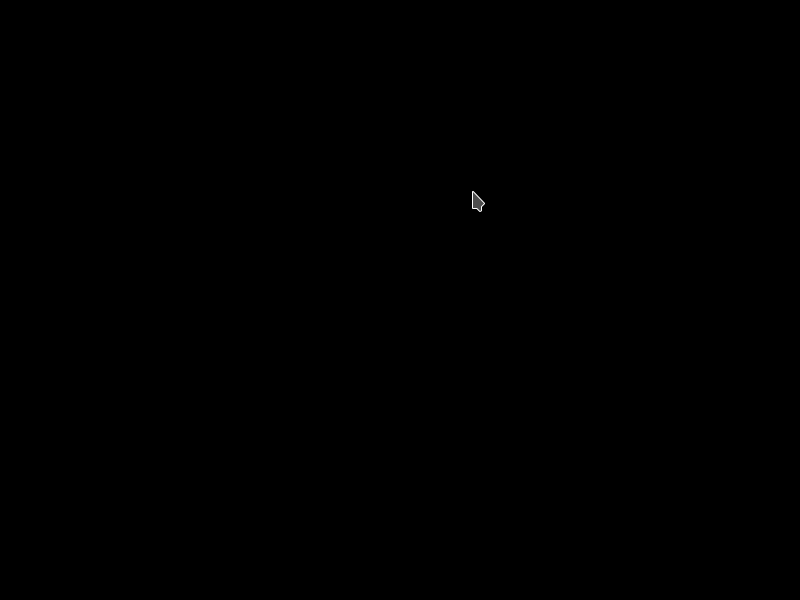
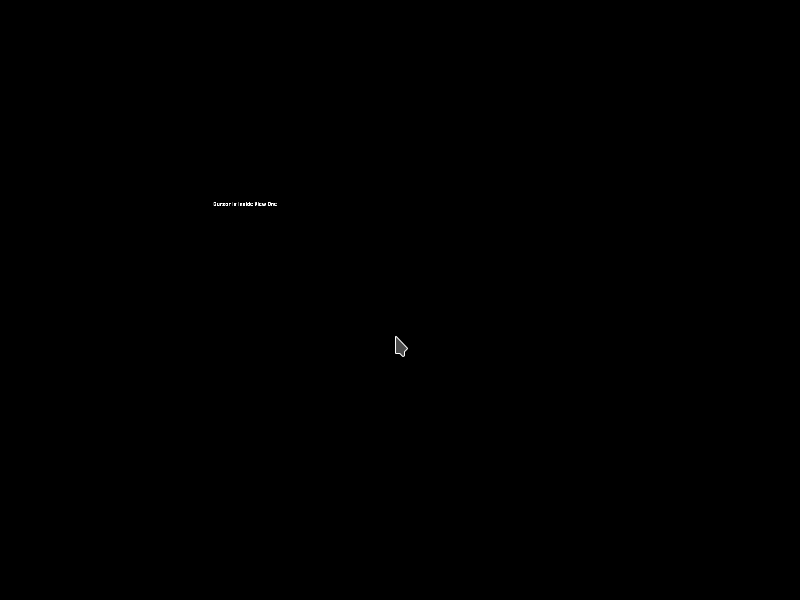
is that supposed to happen? how do I keep the correct proportions, so I can always check if the cursor is inside a given sf::View?
EDIT: I know that the VIEW should be resized, and with it the text inside. but the Viewport should not, I think. notice how the viewport shrinks to almost half the window size in the second image
EDIT 2: it works if I use
view_one.reset(sf::FloatRect(0, 0, 3000, 3000));
is this a bug? I checked the source, and apart from resizing the view (like setSize() does), reset() just recalculates the view center and set the rotation to 0;
7
Graphics / sf::text not handling characters correctly
« on: October 05, 2019, 07:58:24 pm »
hello
I'm having trouble drawing non ASCII character. i searched around, but did not found a good solution.
in the SFML window, I have this:
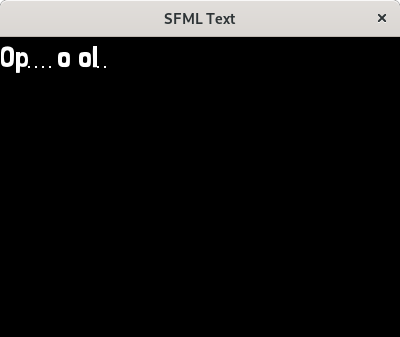
but when I print the same string to the console, it works:

inside the debugger the word is also correct, what makes me think its a problem in SFML interpretation of the string?
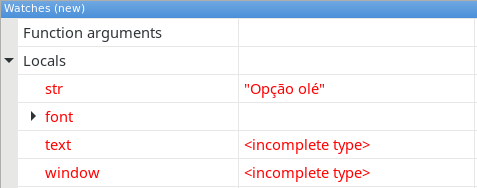
I have read the text tutorial, but I cant understand why just the SFML window is not showing the string correctly.
I tried many font types, of course all of them have the desider characters.
i'm using Linux, by the way.
this is the code:
I also tried changing the sf::Text creation line to
do I have to change every string in my code to wstring? that would be a pain in the neck, mainly because too many functions take just strings as arguments
I'm having trouble drawing non ASCII character. i searched around, but did not found a good solution.
in the SFML window, I have this:
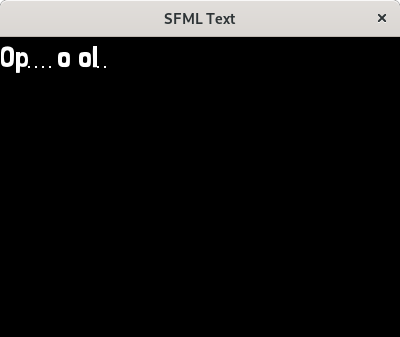
but when I print the same string to the console, it works:

inside the debugger the word is also correct, what makes me think its a problem in SFML interpretation of the string?
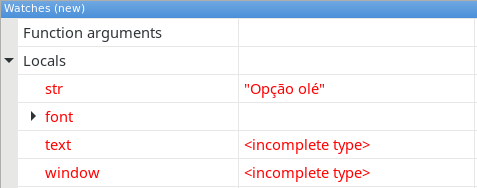
I have read the text tutorial, but I cant understand why just the SFML window is not showing the string correctly.
I tried many font types, of course all of them have the desider characters.
i'm using Linux, by the way.
this is the code:
#include <string>
#include <iostream>
#include <SFML/Graphics/RenderWindow.hpp>
#include <SFML/Window/Event.hpp>
#include <SFML/Graphics/Font.hpp>
#include <SFML/Graphics/Text.hpp>
int main(){
std::string str = "Opção olé";
sf::Font font;
font.loadFromFile("graphics/LondrinaSolid-Regular.otf");
sf::Text text(str, font);
sf::RenderWindow window(sf::VideoMode(400, 300), "SFML Text");
std::cout << str;
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(text);
window.display();
}
return 0;
}
#include <iostream>
#include <SFML/Graphics/RenderWindow.hpp>
#include <SFML/Window/Event.hpp>
#include <SFML/Graphics/Font.hpp>
#include <SFML/Graphics/Text.hpp>
int main(){
std::string str = "Opção olé";
sf::Font font;
font.loadFromFile("graphics/LondrinaSolid-Regular.otf");
sf::Text text(str, font);
sf::RenderWindow window(sf::VideoMode(400, 300), "SFML Text");
std::cout << str;
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(text);
window.display();
}
return 0;
}
I also tried changing the sf::Text creation line to
sf::Text text(sf::String(str), font);
but I have the same results.do I have to change every string in my code to wstring? that would be a pain in the neck, mainly because too many functions take just strings as arguments

8
General / (almost) binary space partitioning example
« on: September 23, 2019, 04:38:02 pm »
hello everybody
I created this small example of BSP... its not done yet, and still a little buggy. but I wanted to share and ask for opinions
i'm planning to use it in some kind of random city creator. you press spacebar and it starts partitioning:
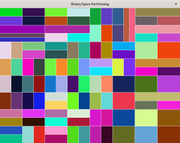
and here goes the code:
(I know most people don't like globals, but its just for testing a prototype...)
=====EDIT=====
changed the code a bit
still produces some weirdness, but its much better. now it have streets
its kind of messy, tough. not intended for real life usage like it is, i guess.
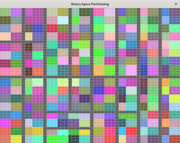
I created this small example of BSP... its not done yet, and still a little buggy. but I wanted to share and ask for opinions
i'm planning to use it in some kind of random city creator. you press spacebar and it starts partitioning:
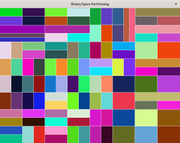
and here goes the code:
(I know most people don't like globals, but its just for testing a prototype...)
(click to show/hide)
=====EDIT=====
changed the code a bit
still produces some weirdness, but its much better. now it have streets

its kind of messy, tough. not intended for real life usage like it is, i guess.
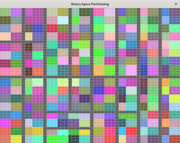
(click to show/hide)
9
Graphics / reset Transform?
« on: July 25, 2018, 02:14:31 pm »
hello everybody
how can I reset a transform? i noticed that if i use
what i'm trying to do is move some vertexArrays to specific positions on the screen, so i can't just combine the "translate" values.
thanks in advance
how can I reset a transform? i noticed that if i use
state.transform.translate = sf::Vector2f(10, 0);
twice, the final position will be (20, 0). i know that it is expected, but, how can i make it to be always (10, 0)?what i'm trying to do is move some vertexArrays to specific positions on the screen, so i can't just combine the "translate" values.
thanks in advance

10
Graphics / positioning sf::Text
« on: May 14, 2018, 01:16:27 pm »
hello everybody
i want to align a text box with the bottom edge of the screen. but the way i'm doing it is not working correctly. this is a minimal code example:
and this is the result:
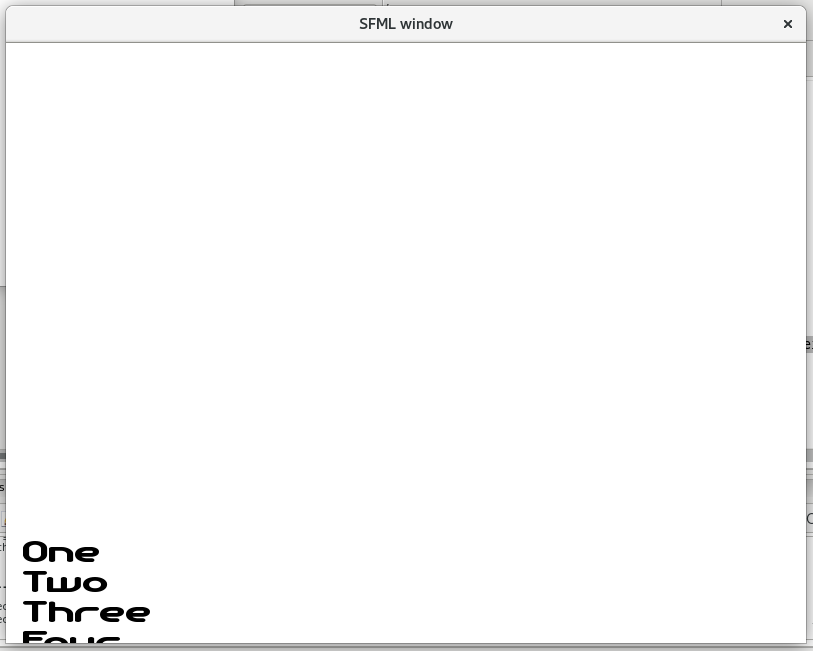
i searched it, but haven't found anything about...
i'm using SFML 2.4 from the oficial Debian Testing repositories.
thanks in advance
i want to align a text box with the bottom edge of the screen. but the way i'm doing it is not working correctly. this is a minimal code example:
#include <SFML/Graphics.hpp>
int main(){
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML window");
sf::Font font;
font.loadFromFile("automati.ttf");
sf::Text text("One\nTwo\nThree\nFour", font, 30);
text.setPosition(window.getSize().x*0.02, window.getSize().y - text.getGlobalBounds().height);
text.setColor(sf::Color::Black);
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed){
window.close();
}
}
window.clear(sf::Color::White);
window.draw(text);
window.display();
}
return 0;
}
int main(){
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML window");
sf::Font font;
font.loadFromFile("automati.ttf");
sf::Text text("One\nTwo\nThree\nFour", font, 30);
text.setPosition(window.getSize().x*0.02, window.getSize().y - text.getGlobalBounds().height);
text.setColor(sf::Color::Black);
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed){
window.close();
}
}
window.clear(sf::Color::White);
window.draw(text);
window.display();
}
return 0;
}
and this is the result:
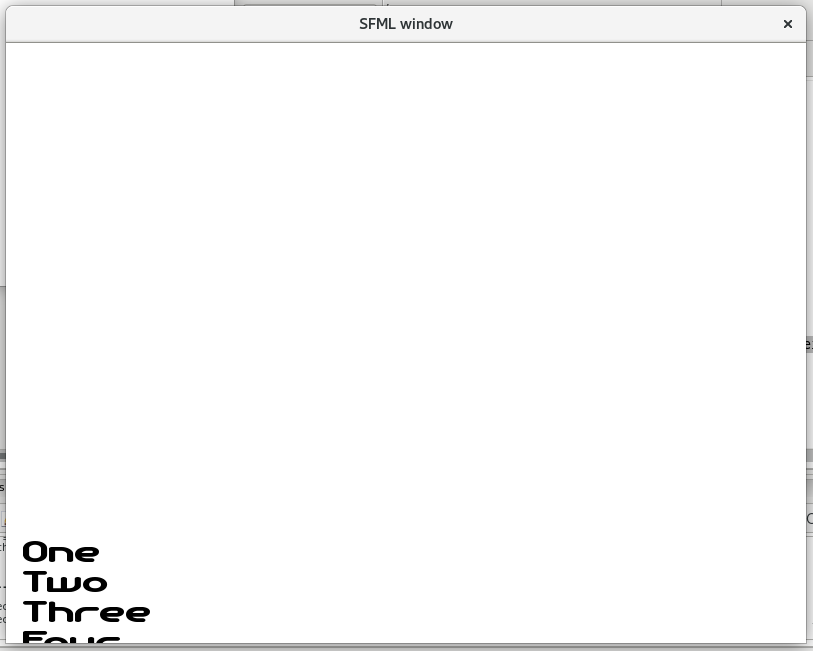
i searched it, but haven't found anything about...
i'm using SFML 2.4 from the oficial Debian Testing repositories.
thanks in advance

11
General / "infinite" tilemap - please rate & help improve
« on: November 10, 2016, 03:07:33 am »
hello everybody.
i created this little snippet of a seamless "infinite" tilemap. it works, but i feel like it could be better. so i'm taking suggestions for everything, even variable names.
how it works:
1- check if player has moved from one tile to another
2- if yes, then search in a binary file for the current player tile
3- load player tile and some tiles around it, just enough to fill a little more than the screen
4- draw the tiles
the code is below (<100 lines). tileset is attached.
and this is the code that generates the binary file that stores the tiles:
I noticed that if you start at some position *relatively close* to negative values (ie 10, 10), it doesn't work as expected. i don't exactly know how to fix it.
let me know if i forgot to explain something. besides that, i take any suggestions. i really want to improve this.
thanks in advance
i created this little snippet of a seamless "infinite" tilemap. it works, but i feel like it could be better. so i'm taking suggestions for everything, even variable names.
how it works:
1- check if player has moved from one tile to another
2- if yes, then search in a binary file for the current player tile
3- load player tile and some tiles around it, just enough to fill a little more than the screen
4- draw the tiles
the code is below (<100 lines). tileset is attached.
(click to show/hide)
and this is the code that generates the binary file that stores the tiles:
(click to show/hide)
I noticed that if you start at some position *relatively close* to negative values (ie 10, 10), it doesn't work as expected. i don't exactly know how to fix it.
let me know if i forgot to explain something. besides that, i take any suggestions. i really want to improve this.
thanks in advance

12
General / a better way to select options from menu?
« on: August 30, 2014, 06:34:49 pm »
hi,
i'd like some suggestions on how to better manage the cursor position on a menu.
for now, i have a enum that holds all options, like
here is an example.
is that a good approach? or is there some better way to make the cursor move on a menu? how do people usually do that?
sorry for this being more a design-related question
thanks in advance!
i'd like some suggestions on how to better manage the cursor position on a menu.
for now, i have a enum that holds all options, like
enum CursorPosition {NEW_GAME, LOAD_GAME, OPTIONS, EXIT);
when the user press DownArrow, the CursorPosition goes from NEW_GAME to LOAD_GAME. if he press it again, the cursor goes to OPTIONS, etc. as its not possible to directly iterate trough enums, i use static_cast. but i feel it's too messy.here is an example.
#include <SFML/Graphics.hpp>
enum Cursor{FIRST, SECOND, THIRD, FOURTH};
int main(){
sf::RenderWindow window(sf::VideoMode(600, 480), "SFML window");
const size_t num_options = 3;
sf::RectangleShape rect[num_options];
for (size_t n=0; n<num_options; n++){
rect[n].setPosition(20, n*100+20);
rect[n].setSize(sf::Vector2f(560, 80));
}
Cursor cursor = FIRST;
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed){
window.close();
}
//////////////////////////////////////////////
//Here is where I handle the cursor movement//
else if (event.type == sf::Event::KeyPressed){
int c = static_cast<int>(cursor);
switch(event.key.code){
case sf::Keyboard::Up:{
c--;
if (c<0){c=0;}
break;
}
case sf::Keyboard::Down:{
c++;
if (c>=num_options){c=num_options-1;}
break;
}
default:{
break;
}
}
cursor = static_cast<Cursor>(c);
}
}
window.clear(sf::Color::White);
for (size_t n=0; n<num_options; n++){
rect[n].setFillColor(sf::Color::Green);
if (cursor == static_cast<Cursor>(n)){
rect[n].setFillColor(sf::Color::Red);
}
window.draw(rect[n]);
}
window.display();
}
return 0;
}
enum Cursor{FIRST, SECOND, THIRD, FOURTH};
int main(){
sf::RenderWindow window(sf::VideoMode(600, 480), "SFML window");
const size_t num_options = 3;
sf::RectangleShape rect[num_options];
for (size_t n=0; n<num_options; n++){
rect[n].setPosition(20, n*100+20);
rect[n].setSize(sf::Vector2f(560, 80));
}
Cursor cursor = FIRST;
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed){
window.close();
}
//////////////////////////////////////////////
//Here is where I handle the cursor movement//
else if (event.type == sf::Event::KeyPressed){
int c = static_cast<int>(cursor);
switch(event.key.code){
case sf::Keyboard::Up:{
c--;
if (c<0){c=0;}
break;
}
case sf::Keyboard::Down:{
c++;
if (c>=num_options){c=num_options-1;}
break;
}
default:{
break;
}
}
cursor = static_cast<Cursor>(c);
}
}
window.clear(sf::Color::White);
for (size_t n=0; n<num_options; n++){
rect[n].setFillColor(sf::Color::Green);
if (cursor == static_cast<Cursor>(n)){
rect[n].setFillColor(sf::Color::Red);
}
window.draw(rect[n]);
}
window.display();
}
return 0;
}
is that a good approach? or is there some better way to make the cursor move on a menu? how do people usually do that?
sorry for this being more a design-related question

thanks in advance!
13
General / opinions: how to handle resource errors?
« on: November 04, 2013, 06:48:29 pm »
hello,
i was thinking, how do people usually handle loading erros in SFML?
i mean, if you make a graphical application, without the console running on background, and it fails to load a font: how are you going to say to the user "hey, i had a problem loading fonts"?
because if there is no terminal, you can't output errors to it; and if there's no sf::Font, you can't show a sf::Text on the screen. so, what would you do?
thanks for the suggestions
i was thinking, how do people usually handle loading erros in SFML?
i mean, if you make a graphical application, without the console running on background, and it fails to load a font: how are you going to say to the user "hey, i had a problem loading fonts"?
because if there is no terminal, you can't output errors to it; and if there's no sf::Font, you can't show a sf::Text on the screen. so, what would you do?
thanks for the suggestions

14
Graphics / Transparent color and RenderTexture
« on: October 22, 2013, 02:56:23 am »
hello everybody. consider this little piece of code:
if you render it, you'll get:

BUT, if you change the line

what makes me think: why this difference? I believe that when setting something to sf::Color::Transparent, it stores black pixels (as sf::Color::Transparent = sf::Color(0, 0, 0, 0)). but these pixels shouldn't be shown at all, since they're transparent, right?
as i don't know if this is expected or is a bug, i tought it would be better to ask here
thanks!
#include <SFML/Graphics.hpp>
int main(){
sf::RenderTexture temp_render;
sf::Texture tex;
sf::Sprite spr;
temp_render.create(200, 200);
temp_render.clear(sf::Color::Transparent);
sf::VertexArray square;
sf::Color color_1(255, 0, 0, 255);
sf::Color color_2(255, 0, 0, 0);
square.setPrimitiveType(sf::PrimitiveType::Quads);
square.append(sf::Vertex(sf::Vector2f(0, 0), color_2));
square.append(sf::Vertex(sf::Vector2f(0, 200), color_2));
square.append(sf::Vertex(sf::Vector2f(200, 200), color_1));
square.append(sf::Vertex(sf::Vector2f(200, 0), color_1));
temp_render.draw(square);
temp_render.display();
tex = temp_render.getTexture();
spr.setTexture(tex);
sf::RenderWindow window(sf::VideoMode(200, 200), "SFML Window");
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed)
window.close();
}
window.clear(sf::Color::White);
window.draw(spr);
window.display();
}
return 0;
}
int main(){
sf::RenderTexture temp_render;
sf::Texture tex;
sf::Sprite spr;
temp_render.create(200, 200);
temp_render.clear(sf::Color::Transparent);
sf::VertexArray square;
sf::Color color_1(255, 0, 0, 255);
sf::Color color_2(255, 0, 0, 0);
square.setPrimitiveType(sf::PrimitiveType::Quads);
square.append(sf::Vertex(sf::Vector2f(0, 0), color_2));
square.append(sf::Vertex(sf::Vector2f(0, 200), color_2));
square.append(sf::Vertex(sf::Vector2f(200, 200), color_1));
square.append(sf::Vertex(sf::Vector2f(200, 0), color_1));
temp_render.draw(square);
temp_render.display();
tex = temp_render.getTexture();
spr.setTexture(tex);
sf::RenderWindow window(sf::VideoMode(200, 200), "SFML Window");
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed)
window.close();
}
window.clear(sf::Color::White);
window.draw(spr);
window.display();
}
return 0;
}
if you render it, you'll get:

BUT, if you change the line
window.draw(spr);
towindow.draw(square);
meaning that you'll draw the square directly to the screen, instead of drawing it to a RenderTexture and then to the screen, you'll get:
what makes me think: why this difference? I believe that when setting something to sf::Color::Transparent, it stores black pixels (as sf::Color::Transparent = sf::Color(0, 0, 0, 0)). but these pixels shouldn't be shown at all, since they're transparent, right?
as i don't know if this is expected or is a bug, i tought it would be better to ask here

thanks!
15
Graphics / how to properly to use this shader?
« on: October 03, 2013, 06:26:31 pm »
hello everybody. i'm trying to create an outline effect for texts in SFML. since it's not natively possible, i'm trying to use a shader for that, which is found here.
so, i pasted it in a file called outline.shader, and load it in the code like this:
and theeen, i get this completely weird result:

i don't have any experience with shaders, so i barely understand what's happening for this strange result shows up.
so, could anyone help me solve this?
thanks in advance!
so, i pasted it in a file called outline.shader, and load it in the code like this:
if(sf::Shader::isAvailable()){
std::cout << "Shaders are avaliable\n";
text_outline.loadFromFile("images/fonts/outline.shader", sf::Shader::Fragment);
text_outline.setParameter("outlineColor", sf::Color::White);
text_outline.setParameter("texSize", sf::Vector2f(2.0, 2.0));
}
[...]
game_window.draw(title_text, &text_outline);
std::cout << "Shaders are avaliable\n";
text_outline.loadFromFile("images/fonts/outline.shader", sf::Shader::Fragment);
text_outline.setParameter("outlineColor", sf::Color::White);
text_outline.setParameter("texSize", sf::Vector2f(2.0, 2.0));
}
[...]
game_window.draw(title_text, &text_outline);
and theeen, i get this completely weird result:

i don't have any experience with shaders, so i barely understand what's happening for this strange result shows up.
so, could anyone help me solve this?
thanks in advance!
Pages: [1] 2