1
SFML projects / My current project using SFML
« on: January 25, 2013, 09:27:25 am »
Hello!
Wanted to share the current project I am working on entitled The Dungeon 2: The Key of Antioch. Its a sequel to the first Dungeon, and the demo is really just a playable mini game of an aspect of the whole project. Since I hit a milestone I thought it might be cool to whip up a little game and demonstrate what I have been working on.
So...
Take a gander at my web page, and download!
Wanted to share the current project I am working on entitled The Dungeon 2: The Key of Antioch. Its a sequel to the first Dungeon, and the demo is really just a playable mini game of an aspect of the whole project. Since I hit a milestone I thought it might be cool to whip up a little game and demonstrate what I have been working on.
So...
Take a gander at my web page, and download!
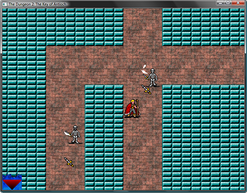