Using SFML 2.1, Code::Blocks 12.11, minGW gcc-4.7.1-tdm, "ThinkPad Display 1366x768" monitorMy issue is how a certain sprite looks by default when generated on the screen.
Here's the necessary code:
const int WINDOW_WIDTH = 1024;
const int WINDOW_HEIGHT = 576;
sf::RenderWindow Window;
Window.create(sf::VideoMode(WINDOW_WIDTH, WINDOW_HEIGHT), "azertyqwerty", sf::Style::Close);
sf::Texture playerTexture;
sf::Sprite playerSprite;
if (!playerTexture.loadFromFile("player.png"))
std::cout << "Error: Could not load player image" << std::endl;
playerSprite.setTexture(playerTexture);
playerSprite.setOrigin(playerSprite.getLocalBounds().width/2, playerSprite.getLocalBounds().height/2);
playerSprite.setPosition(WINDOW_WIDTH/2, WINDOW_HEIGHT/2);
while (Window.isOpen())
{
Window.clear();
Window.draw(playerSprite);
Window.display();
}
Here's the original image file used:
http://i1093.photobucket.com/albums/i434/GanadoFO/player.png
It is a
5x5 square image. The border is red and the center pixel is a golden color.
Here's what the sprite looks like when displayed, no scaling or other transformations:

Here's the same picture enlarged (externally in Paint, not via the scale function) to clearly show the error:
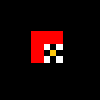
You can see the weird displacement of the pixels compared to the original image. This is the problem.
If I use the scale function to blow-up the image in program run-time, it becomes the intended look, albeit very big:
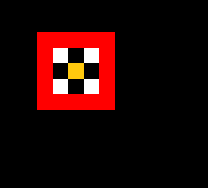
So, my questions:
Why does it not correctly display the image file the way it is? Why does it displace the border pixels, and how can I fix it?
Is it due to SFML itself or my monitor/gpu?
If someone can test my image to see if it does the same thing on their program, I would appreciate it, thanks.