Hi, I've been having problems getting a wxWidgets-integrated RenderWindow to behave correctly in linux/gtk. Basically it seems that rendering anything that relies on the current RenderWindow view will not work. It's as if the RenderWindow ignores the size of the wx control itself and just sets the view to a small 14x14 square on the bottom left corner of the control, rather than using the full space.
I've written a small example program below:
#include <wx/wx.h>
#include <SFML/Graphics.hpp>
#ifdef __WXGTK__
#include <gdk/gdkx.h>
#include <gtk/gtk.h>
#include <gdk/gdkprivate.h>
#include <gtk/gtkwidget.h>
#endif
class SFMLRenderWindow : public wxControl, public sf::RenderWindow {
public:
SFMLRenderWindow(wxWindow* parent, int id) : wxControl(parent, id) {
#ifdef __WXGTK__
gtk_widget_realize(m_wxwindow);
gtk_widget_set_double_buffered(m_wxwindow, false);
GdkWindow* Win = gtk_widget_get_window(m_wxwindow);
XFlush(GDK_WINDOW_XDISPLAY(Win));
sf::RenderWindow::Create(GDK_WINDOW_XWINDOW(Win));
#else
sf::RenderWindow::Create(GetHandle());
#endif
Bind(wxEVT_PAINT, &SFMLRenderWindow::onPaint, this);
Bind(wxEVT_ERASE_BACKGROUND, &SFMLRenderWindow::onEraseBackground, this);
Bind(wxEVT_IDLE, &SFMLRenderWindow::onIdle, this);
Bind(wxEVT_KEY_DOWN, &SFMLRenderWindow::onKeyDown, this);
}
~SFMLRenderWindow() {}
void onPaint(wxPaintEvent& e) {
wxPaintDC(this);
sf::RenderWindow::SetActive();
Clear(sf::Color(0,0,100));
sf::View& view = GetDefaultView();
view.SetFromRect(sf::FloatRect(0.0f, 0.0f, (float)GetSize().x, (float)GetSize().y));
sf::String str("Testing 123");
Draw(str);
Display();
}
void onEraseBackground(wxEraseEvent& e) {
// Do nothing
}
void onIdle(wxIdleEvent& e) {
if (GetFrameTime() > 0.02f)
Refresh();
}
void onKeyDown(wxKeyEvent& e) {
if (e.GetKeyCode() == WXK_F11) {
sf::Image image = Capture();
image.SaveToFile("out.png");
}
else
e.Skip();
}
};
// Test application
class wxMiniApp : public wxApp {
public:
virtual bool OnInit();
};
IMPLEMENT_APP(wxMiniApp)
bool wxMiniApp::OnInit() {
wxFrame* frame = new wxFrame(NULL, -1, "SFML RenderWindow", wxDefaultPosition, wxSize(800, 600));
new SFMLRenderWindow(frame, -1);
frame->Show();
return true;
}
This works as expected in windows - "Testing 123" in the default font is rendered in the top left of the window, over a blue background.
In linux, though, I get this:
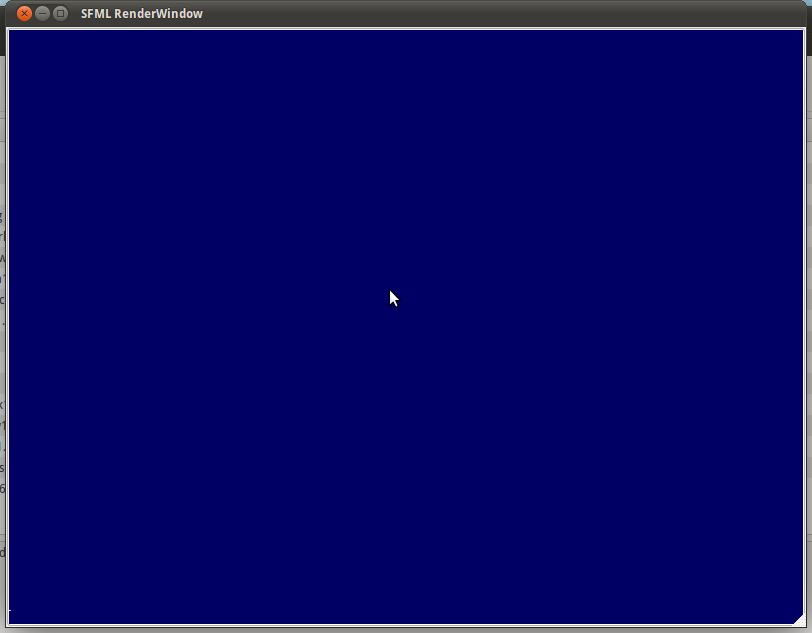
(notice the white pixel at the lower left)
The output from the screenshot button is this:

which is obviously wrong.
I'm not sure what is going on with it, and using RenderWindow::SetSize makes no difference at all. I can draw on the full window using custom opengl commands and setting up the projection matrix myself, but trying to use any of the SFML Drawables won't work.
Versions of libraries/systems in question:
Ubuntu 11.04 x64
wxWidgets 2.9.2 svn
SFML 1.6 (tried 2.0 as well, but that did the same thing)