So after getting SFML 1.6 working, I find out that people on the project I'm working on can't get 1.6 working but can get 2.0 working. So I follow the tutorial that explains how to create the VS project files using CMake. I tried both VS 2010 and VS2005 (changing the settings in CMake accordingly) and both gave me the same behaviour when I tried to link statically to the libraries:
Error 1 error LNK2019: unresolved external symbol "__declspec(dllimport) public: virtual __thiscall sf::Text::~Text(void)" (__imp_??1Text@sf@@UAE@XZ) referenced in function _main main.obj
Error 2 error LNK2019: unresolved external symbol "__declspec(dllimport) public: void __thiscall sf::Window::Display(void)" (__imp_?Display@Window@sf@@QAEXXZ) referenced in function _main main.obj
Error 3 error LNK2019: unresolved external symbol "__declspec(dllimport) public: void __thiscall sf::RenderTarget::Draw(class sf::Drawable const &)" (__imp_?Draw@RenderTarget@sf@@QAEXABVDrawable@2@@Z) referenced in function _main main.obj
Error 4 error LNK2019: unresolved external symbol "__declspec(dllimport) public: void __thiscall sf::RenderTarget::Clear(class sf::Color const &)" (__imp_?Clear@RenderTarget@sf@@QAEXABVColor@2@@Z) referenced in function _main main.obj
Error 5 error LNK2019: unresolved external symbol "__declspec(dllimport) public: __thiscall sf::Color::Color(unsigned char,unsigned char,unsigned char,unsigned char)" (__imp_??0Color@sf@@QAE@EEEE@Z) referenced in function _main main.obj
Error 6 error LNK2019: unresolved external symbol "__declspec(dllimport) public: void __thiscall sf::Window::Close(void)" (__imp_?Close@Window@sf@@QAEXXZ) referenced in function _main main.obj
Error 7 error LNK2019: unresolved external symbol "__declspec(dllimport) public: bool __thiscall sf::Window::PollEvent(class sf::Event &)" (__imp_?PollEvent@Window@sf@@QAE_NAAVEvent@2@@Z) referenced in function _main main.obj
Error 8 error LNK2019: unresolved external symbol "__declspec(dllimport) public: bool __thiscall sf::Window::IsOpened(void)const " (__imp_?IsOpened@Window@sf@@QBE_NXZ) referenced in function _main main.obj
Error 9 error LNK2019: unresolved external symbol "__declspec(dllimport) public: __thiscall sf::String::~String(void)" (__imp_??1String@sf@@QAE@XZ) referenced in function _main main.obj
Error 10 error LNK2019: unresolved external symbol "__declspec(dllimport) public: __thiscall sf::Text::Text(class sf::String const &,class sf::Font const &,unsigned int)" (__imp_??0Text@sf@@QAE@ABVString@1@ABVFont@1@I@Z) referenced in function _main main.obj
Error 11 error LNK2019: unresolved external symbol "__declspec(dllimport) public: __thiscall sf::String::String(char const *,class std::locale const &)" (__imp_??0String@sf@@QAE@PBDABVlocale@std@@@Z) referenced in function _main main.obj
Error 12 error LNK2019: unresolved external symbol "__declspec(dllimport) public: virtual __thiscall sf::RenderWindow::~RenderWindow(void)" (__imp_??1RenderWindow@sf@@UAE@XZ) referenced in function _main main.obj
Error 13 error LNK2019: unresolved external symbol "__declspec(dllimport) public: __thiscall sf::Font::~Font(void)" (__imp_??1Font@sf@@QAE@XZ) referenced in function _main main.obj
Error 14 error LNK2019: unresolved external symbol "__declspec(dllimport) public: bool __thiscall sf::Font::LoadFromFile(class std::basic_string<char,struct std::char_traits<char>,class std::allocator<char> > const &)" (__imp_?LoadFromFile@Font@sf@@QAE_NABV?$basic_string@DU?$char_traits@D@std@@V?$allocator@D@2@@std@@@Z) referenced in function _main main.obj
Error 15 error LNK2019: unresolved external symbol "__declspec(dllimport) public: __thiscall sf::Font::Font(void)" (__imp_??0Font@sf@@QAE@XZ) referenced in function _main main.obj
Error 16 error LNK2019: unresolved external symbol "__declspec(dllimport) public: __thiscall sf::RenderWindow::RenderWindow(class sf::VideoMode,class std::basic_string<char,struct std::char_traits<char>,class std::allocator<char> > const &,unsigned long,struct sf::ContextSettings const &)" (__imp_??0RenderWindow@sf@@QAE@VVideoMode@1@ABV?$basic_string@DU?$char_traits@D@std@@V?$allocator@D@2@@std@@KABUContextSettings@1@@Z) referenced in function _main main.obj
Error 17 error LNK2019: unresolved external symbol "__declspec(dllimport) public: __thiscall sf::VideoMode::VideoMode(unsigned int,unsigned int,unsigned int)" (__imp_??0VideoMode@sf@@QAE@III@Z) referenced in function _main main.obj
Error 18 fatal error LNK1120: 17 unresolved externals E:\Dev\Projects\VS2005 SFML2.0 Test\Debug\VS2005 SFML2.0 Test.exe
I can see that those errors look like I haven't included the correct libraries, but I've checked it a dozen times and it's all right.
The code I compiled with both IDEs:
#include "SFML/Audio.hpp"
#include "SFML/Graphics.hpp"
int main()
{
// Create the main window
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML window");
sf::Font font;
if(!font.LoadFromFile("arial.ttf"))
{
return EXIT_FAILURE;
}
sf::Text text("hello sfml", font, 50);
// Start the game loop
while (window.IsOpened())
{
// Process events
sf::Event event;
while (window.PollEvent(event))
{
// Close window : exit
if (event.Type == sf::Event::Closed)
window.Close();
}
// Clear screen
window.Clear();
window.Draw(text);
// Update the window
window.Display();
}
return EXIT_SUCCESS;
}
VS2005 debug C++ settings:
/Od /I "E:\Dev\SFML-2.0\include" /D "WIN32" /D "_DEBUG" /D "_CONSOLE" /D "_MBCS" /Gm /EHsc /RTC1 /MTd /Fo"Debug\\" /Fd"Debug\vc80.pdb" /W3 /nologo /c /ZI /TP /errorReport:prompt
VS2005 debug (static) Linker settings:
/OUT:"E:\Dev\Projects\VS2005 SFML2.0 Test\Debug\VS2005 SFML2.0 Test.exe" /INCREMENTAL /NOLOGO /LIBPATH:"E:\Dev\SFML-2.0\8build\lib\debug" /MANIFEST /MANIFESTFILE:"Debug\VS2005 SFML2.0 Test.exe.intermediate.manifest" /DEBUG /PDB:"e:\dev\projects\vs2005 sfml2.0 test\debug\VS2005 SFML2.0 Test.pdb" /SUBSYSTEM:CONSOLE /MACHINE:X86 /ERRORREPORT:PROMPT sfml-audio-s-d.lib sfml-system-s-d.lib sfml-window-s-d.lib sfml-graphics-s-d.lib kernel32.lib user32.lib gdi32.lib winspool.lib comdlg32.lib advapi32.lib shell32.lib ole32.lib oleaut32.lib uuid.lib odbc32.lib odbccp32.lib
VS2005 release libs:
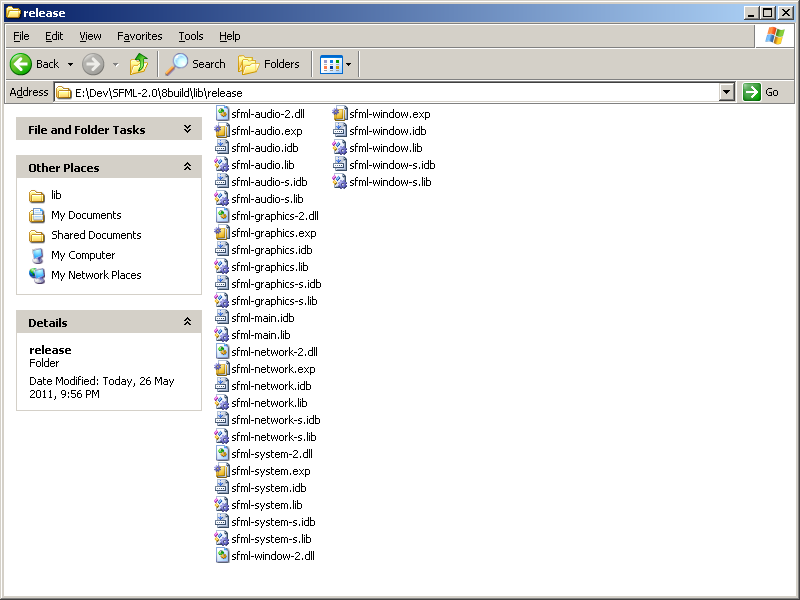
VS2005 debug libs:
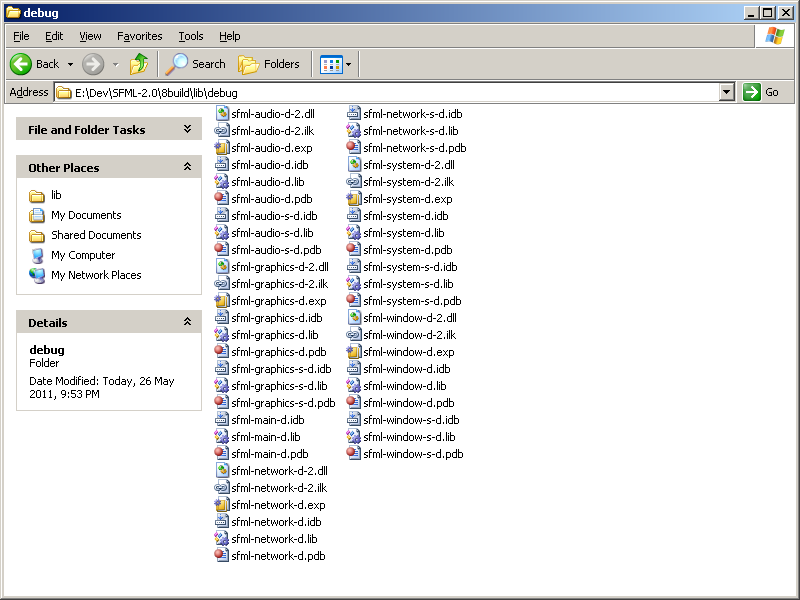
VS2010 (all libs):
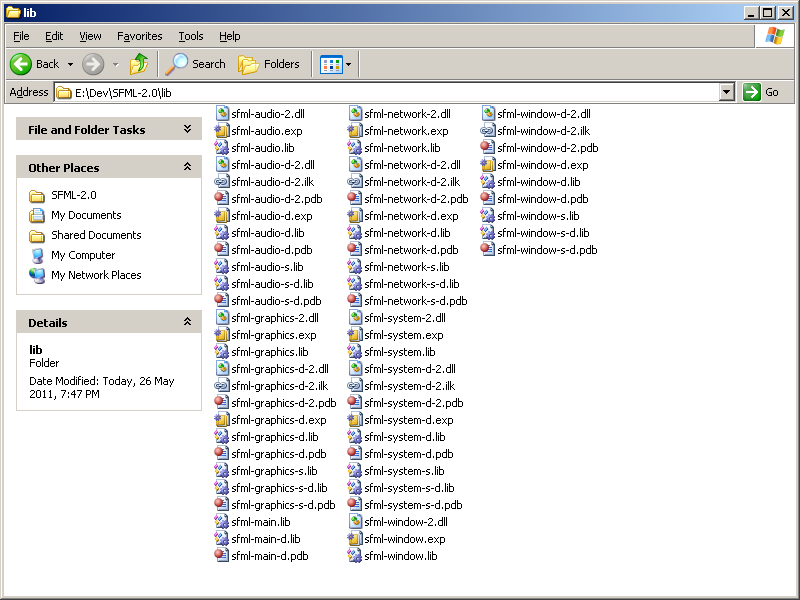
So I tried to link dynamically...
VS2010 debug C++ settings:
/I"E:\Dev\SFML-2.0\include" /ZI /nologo /W3 /WX- /Od /Oy- /D "WIN32" /D "_DEBUG" /D "_CONSOLE" /D "_MBCS" /Gm /EHsc /RTC1 /MDd /GS /fp:precise /Zc:wchar_t /Zc:forScope /Fp"Debug\VS2010 SFM 2.0 Test.pch" /Fa"Debug\" /Fo"Debug\" /Fd"Debug\vc100.pdb" /Gd /analyze- /errorReport:queue
VS2010 debug (static) Linker settings:
/OUT:"E:\Dev\Projects\VS2010 SFM 2.0 Test\Debug\VS2010 SFM 2.0 Test.exe" /INCREMENTAL /NOLOGO /LIBPATH:"E:\Dev\SFML-2.0\lib" "sfml-graphics-d.lib" "sfml-audio-d.lib" "sfml-window-d.lib" "sfml-system-d.lib" "kernel32.lib" "user32.lib" "gdi32.lib" "winspool.lib" "comdlg32.lib" "advapi32.lib" "shell32.lib" "ole32.lib" "oleaut32.lib" "uuid.lib" "odbc32.lib" "odbccp32.lib" /MANIFEST /ManifestFile:"Debug\VS2010 SFM 2.0 Test.exe.intermediate.manifest" /ALLOWISOLATION /MANIFESTUAC:"level='asInvoker' uiAccess='false'" /DEBUG /PDB:"E:\Dev\Projects\VS2010 SFM 2.0 Test\Debug\VS2010 SFM 2.0 Test.pdb" /SUBSYSTEM:CONSOLE /PGD:"E:\Dev\Projects\VS2010 SFM 2.0 Test\Debug\VS2010 SFM 2.0 Test.pgd" /TLBID:1 /DYNAMICBASE /NXCOMPAT /MACHINE:X86 /ERRORREPORT:QUEUE
It compiles fine, but gives me this error:
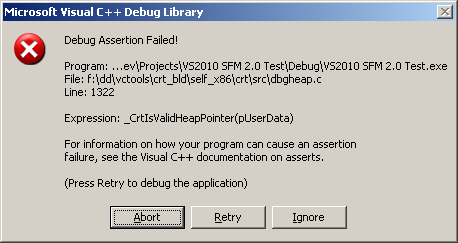
Call stack (pointing to the construction of the sf::Text object):
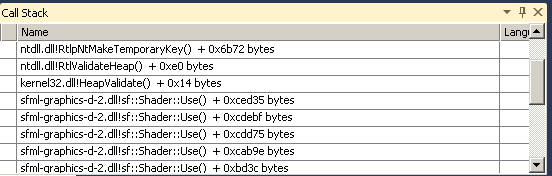
Any help would be greatly appreciated.
Cheers.