Hi !
I'm using the SFML 2.1 library for a game project, my project needs to use an A* algorithm to guide a character on the map to avoid obstacles. I'm using too the Thor library (
http://www.bromeon.ch/libraries/thor/) to help me for the triangulation, indeed I want to use the triangulation to create a navigation mesh in the goal to ease the task of the A* algorithm. A little bit like this :
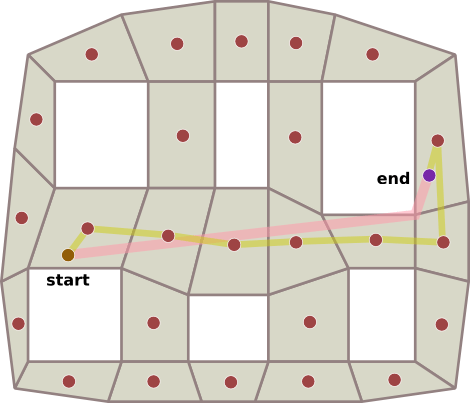
The blank polygon represents an obstacle
I work with the Thor library to have a similar result,

The black areas represents obstacles
1) I generate 4 points which represent a rectangle (it may be polygon later), I put these points in a vertex container and I put the 2 triangles which compose the obstacle in an obstacle container.
if (mouseEvent.button == sf::Mouse::Left)
{
AURORA_CITR_FOREACH(itr, _vertexContainer)
{
if (*itr == clickPos) // Don't insert the same obstacle twice
return false;
}
_vertexContainer.push_back(*pt1); // insert the 4 points of the obstacle (which is a rectangle for now)
_vertexContainer.push_back(*pt2);
_vertexContainer.push_back(*pt3);
_vertexContainer.push_back(*pt4);
Obstacle triangle1 (pt1, pt2, pt3);
Obstacle triangle2 (pt1, pt4, pt3);
_obstacles.push_back(triangle1);
_obstacles.push_back(triangle2);
return true;
}
2) I compute new triangulation for points of the vertex container
this->_triangleContainer.clear();
thor::triangulate(_vertexContainer.begin(), _vertexContainer.end(), std::back_inserter(_triangleContainer));
I run throught my obstacle container and through my triangle container and I remove the triangles that are part of obstacles.
std::vector<Obstacle>::iterator it = _obstacles.begin();
std::vector<thor::Triangle<sf::Vector2f>>::iterator it2 = _triangleContainer.begin();
while (it != _obstacles.end())
{
it2 = _triangleContainer.begin();
while (it2 != _triangleContainer.end())
{
if ((*it2)[0] == *(*it)._pt2 && (*it2)[1] == *(*it)._pt1 && (*it2)[2] == *(*it)._pt3) // I compare the obstacle's points with the triangle's points
{
it2 = _triangleContainer.erase(it2);
}
else if ((*it2)[0] == *(*it)._pt3 && (*it2)[1] == *(*it)._pt1 && (*it2)[2] == *(*it)._pt2)
it2 = _triangleContainer.erase(it2);
else if ((*it2)[0] == *(*it)._pt1 && (*it2)[1] == *(*it)._pt3 && (*it2)[2] == *(*it)._pt2)
it2 = _triangleContainer.erase(it2);
else if ((*it2)[0] == *(*it)._pt1 && (*it2)[1] == *(*it)._pt2 && (*it2)[2] == *(*it)._pt3)
it2 = _triangleContainer.erase(it2);
else if ((*it2)[0] == *(*it)._pt3 && (*it2)[1] == *(*it)._pt2 && (*it2)[2] == *(*it)._pt1)
it2 = _triangleContainer.erase(it2);
else
++it2;
}
++it;
}
At least I run A* algorithm with the different points (ends and midpoints of the sides of triangles).
But as you can see this is not optimized, and I don't know what is the better way to use the triangulation algorithm as navigation mesh. For now my code works with rectangles, but it doesn't work with polygons.... Could you give me some advices about this ?
Thank you in advance.