By debug-mode, do you mean this:
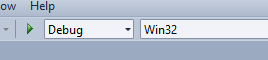
And by release libs, are they under linker > additional dependencies?
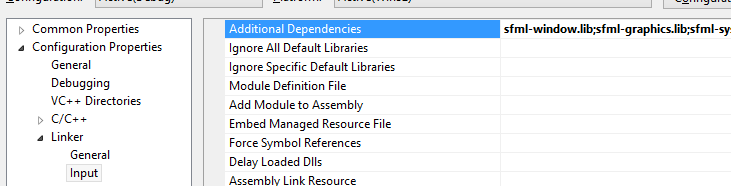
I tried to change each name to add a -d for debug, but the error persists. I am now trying from scratch with the arial font from Windows/fonts.
#include <SFML/Graphics.hpp>
#include <iostream>
int main () {
sf::Font font;
if (!font.loadFromFile("arial.ttf"))
{
std::cout << "Load Failed!" <<std::endl;
return 0;
}
sf::RenderWindow window(sf::VideoMode(200, 200), "SFML Text Test!");
sf::Text text;
// select the font
text.setFont(font); // font is a sf::Font
// set the string to display
text.setString("Hello world");
// set the character size
text.setCharacterSize(24); // in pixels, not points!
// set the color
text.setColor(sf::Color::Red);
// set the text style
text.setStyle(sf::Text::Bold | sf::Text::Underlined);
while (window.isOpen())
{
sf::Event event;
while (window.pollEvent(event))
{
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(text);
window.display();
}
return 0;
}
It runs into that error before even going into the failure code.