Subject of the issueThings are not drawn at the positions they are supposed to in high DPI systems.
Since this problem does not happen when I am testing it on my low resolution PC (1680x1050), it seems to be DPI-related.
Environment- System: Windows 10 home edition version 1703 build 15063.608
- System type: 64bit
- Version of SFML: 2.5.1 for VS2013-32bit and VS2017-32bit (from https://www.sfml-dev.org/download/sfml/2.5.1/)
- Visual Studio version: VS2013 update5, VS2017
- Configuration: Debug, Release
- Resolution: 1920x1080
Steps to reproduceThe testing code should draw 4 triangles whose top-left vertex are at (100, 100), (100, 600), (600, 100), (600, 600).
#include <SFML/Window.hpp>
#include <SFML/Graphics.hpp>
#include <cstdio>
void appen_triangle(sf::VertexArray& data, float x0, float y0, float dx, float dy, sf::Color color = sf::Color::White) {
data.append(sf::Vertex({ x0, y0 }, color));
data.append(sf::Vertex({ x0 + dx, y0 }, color));
data.append(sf::Vertex({ x0, y0 + dy }, color));
}
int main() {
// create the window
int w_width = 800, w_height = 800;
sf::ContextSettings settings(0U, 0U, 4);
sf::RenderWindow window(sf::VideoMode(w_width, w_height), "Test", sf::Style::Default, settings);
window.setView(sf::View(sf::FloatRect(0.0f, 0.0f, (float)w_width, (float)w_height)));
sf::VertexArray data;
data.setPrimitiveType(sf::PrimitiveType::Triangles);
appen_triangle(data, 100.0f, 100.0f, 100.0f, 100.0f);
appen_triangle(data, 600.0f, 100.0f, 100.0f, 100.0f);
appen_triangle(data, 100.0f, 600.0f, 100.0f, 100.0f);
appen_triangle(data, 600.0f, 600.0f, 100.0f, 100.0f);
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed) {
window.close();
}
else if (event.type == sf::Event::MouseButtonPressed) {
printf("Mouse, by pixel = (%d, %d), ", event.mouseButton.x, event.mouseButton.y);
auto p = window.mapPixelToCoords({ event.mouseButton.x, event.mouseButton.y });
printf("by coords=(%.2f, %.2f)\n", p.x, p.y);
}
}
window.clear(sf::Color::Black);
window.draw(data);
window.display();
}
return 0;
}
Expected behavior4 triangles whose top-left vertex are at (100, 100), (100, 600), (600, 100), (600, 600).
Actual behaviorScreenshot of the program.
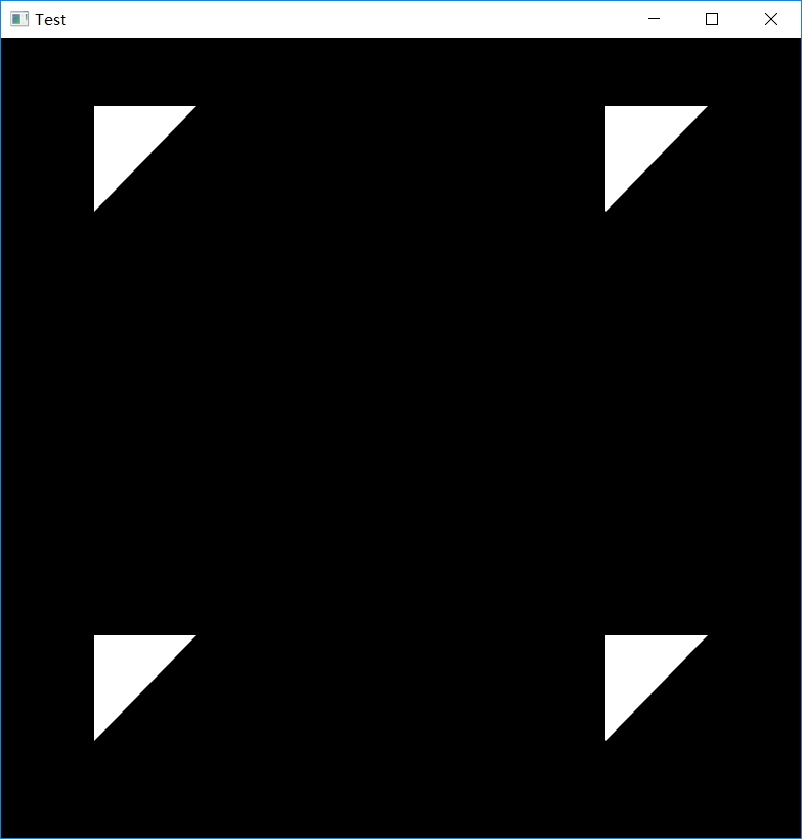
I clicked at each of the top-left vertex of the triangles and the coordinates printed by the program are as follows (Top-Left, Top-Right, Bottom-Left, Bottom-Right).
Mouse, by pixel = (95, 70), by coords=(95.00, 70.00)
Mouse, by pixel = (605, 69), by coords=(605.00, 69.00)
Mouse, by pixel = (94, 600), by coords=(94.00, 600.00)
Mouse, by pixel = (607, 599), by coords=(607.00, 599.00)
Examining the screenshot, assume the top-left corner is (1, 1), the pixel coordinates of the top-left vertex of the triangles are as follows (Top-Left, Top-Right, Bottom-Left, Bottom-Right).
94 69
605 69
94 598
605 598
This suggest that the pixel coordinates returned by SFML are correct but somehow vertices are not drawn at positions they're supposed to.
EditMany thanks to FRex.
Something I tried.
- Run the test program on a high DPI Windows 10 build 1803 laptop. Things good.
- Update my laptop to Windows 10 build 1803. Problem remained.
- Run the test program using the Nvidia graphic card. Problem solved.
- Run the test program using the Intel HD graphic card (default). Problem remained.
- Update my Intel HD graphic card driver. Problem solved.
Conclusion:
- Should be a problem with the Intel graphic card driver.
- The system build version may or may not have an influence on this.
Some other information.
- CPU: Intel Core i7-7700HQ
- GPU: Intel HD Graphics 630
- Version of the old driver that causes problems: 21.20.16.4664
- Version of the new driver: 23.20.16.4973