UPDATE: Sorry for the double post, but here's an update. I took Jesper's suggestion and looked on how to create the circular path using the circle equations he linked above. For any point on the circumference,
x = a + r(cos t)
y = b + r(sin t)where
(x,y) is the point,
(a,b) is the center,
t is the angle with X-axis and
r is the radius.
I used these equations and based on them, I wrote the code to draw the nodes on the circular path. And it worked like a charm.

Here's the code if anyone else wants to do anything similar:
Window.clear(sf::Color(255, 255, 255));
std::vector<sf::CircleShape> Circles;
sf::CircleShape Circle(30);
int n = 10, k = 1;
float X, Y;
for(int i = 0; i < n; i++)
{
Circles.push_back(Circle);
}
for(std::vector<sf::CircleShape>::iterator it = Circles.begin(); it != Circles.end(); it++)
{
it->setFillColor(sf::Color(0, 0, 0));
X = 200.0f + 100 * (float)cos(k * (2 * PI/n));
Y = 200.0f + 100 * (float)sin(k * (2 * PI/n));
it->setOrigin(it->getGlobalBounds().width/2, it->getGlobalBounds().height/2);
it->setPosition(X, Y);
Window.draw(*it);
k++;
}
I know the code is very crude and very messy. But the logic behind it is the same. I only did that to test how the output came. It's better to use loops for many nodes. EDIT: I changed the code to make it more optimal with the help of for loops and STL containers such as vectors. The window size I took was 400x400 so the center is (200, 200). Here's what I got for three nodes and four nodes.

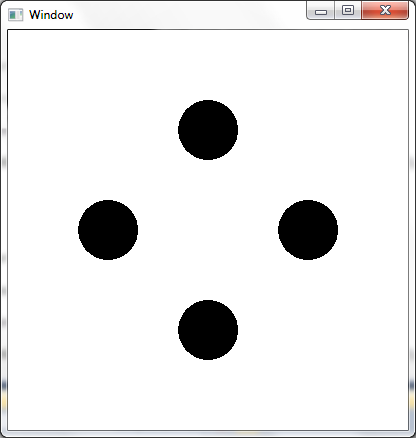
Pretty neat, right? At least it serves my purpose. Thanks,
Jesper Juhl and
eXpl0it3r! For now, I am satisfied with what I got, but please don't close the topic yet. If I hit any more roadblocks with this problem again, I shall ask again. Thank you. Have a nice day!
