Am intently following the vertex-array tutorial on the website, am fixing the algorithm to sort my tiles isometrically. My tiles appear to draw good, but on closer inspection the pixels are sliiightly off. Not so sure what in my algorithm is causing it to be off, I've tried toying with it for several hours with no luck.
Here is an image of the result, including a zoomed/enhanced image of the alignment.
Tiles are exactly 34x18 (WIDTHxHEIGHT) chosen randomly from this tileset:
The load function is correctly taking the appropriate arguments:
tileMap.load("data/gfx/tiles/1.png", sf::Vector2u(34, 18), map, 16); // map is the std::map which contains a std::pair as the key to access the map data at a coordinate.
bool load(const std::string& tileset, sf::Vector2u tileSize, std::map<std::pair<int, int>, int> map, unsigned int size)
{
// to ensure map width/height are the same height
unsigned int width = size, height = size;
// load the tileset texture
if (!m_tileset.loadFromFile(tileset))
return false;
// resize the vertex array to fit the level size
m_vertices.setPrimitiveType(sf::Quads);
m_vertices.resize(width * height * 4);
// Offset to draw more toward the center of the screen, otherwise stuff gets cut off.
int offset_x = ((width/2) * tileSize.x) * 1.2;
int offset_y = (height/2) * 1.2;
for (unsigned int i = 0; i < width; ++i)
{
for (unsigned int j = 0; j < height; ++j)
{
int tileNumber = map[std::make_pair(i, j)];
int tu = tileNumber % (m_tileset.getSize().x / tileSize.x);
int tv = tileNumber / (m_tileset.getSize().x / tileSize.x);
sf::Vertex* quad = &m_vertices[(i + j * width) * 4];
quad[0].position = sf::Vector2f(offset_x + (j * tileSize.x / 2) - (i * tileSize.x / 2), offset_y + (i * tileSize.y / 2) + (j * tileSize.y / 2));
quad[1].position = sf::Vector2f(offset_x + (((j+2) * tileSize.x) / 2) - (i * tileSize.x / 2), offset_y + (i * tileSize.y / 2) + (j * tileSize.y / 2));
quad[2].position = sf::Vector2f(offset_x + (((j+2) * tileSize.x) / 2) - (i * tileSize.x / 2), offset_y + (((i+2) * tileSize.y) / 2) + (j * tileSize.y / 2));
quad[3].position = sf::Vector2f(offset_x + (j * tileSize.x / 2) - (i * tileSize.x / 2), offset_y + (((i+2) * tileSize.y) / 2) + (j * tileSize.y / 2));
quad[0].texCoords = sf::Vector2f(tu * tileSize.x, tv * tileSize.y);
quad[1].texCoords = sf::Vector2f((tu + 1) * tileSize.x, tv * tileSize.y);
quad[2].texCoords = sf::Vector2f((tu + 1) * tileSize.x, (tv + 1) * tileSize.y);
quad[3].texCoords = sf::Vector2f(tu * tileSize.x, (tv + 1) * tileSize.y);
}
}
return true;
}
Any help is greatly appreciated!
Edit: Am using a modified version of this formula I found online which achieves this projection:
tile_map[][] = [[...],...]
for (i = 0; i < tile_map.size; i++):
for (j = tile_map[i].size; j >= 0; j--): // Changed loop condition here.
draw(
tile_map[i][j],
x = (j * tile_width / 2) + (i * tile_width / 2)
y = (i * tile_height / 2) - (j * tile_height / 2)
)
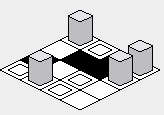