Hello,
I don't want to make a post for every question, maybe i should?
Here are all the questions i encountered so far, if someone could enlighten.. :
1) i reread almost all graphics tutorials except the "view" one, and i did not find a single example of a use of the a Transformable class with a matrix, i saw this on the tutorial page (
https://www.sfml-dev.org/tutorials/2.5/graphics-transform.php)
sf::Transform t3(2.f, 0.f, 20.f,
0.f, 1.f, 50.f,
0.f, 0.f, 1.f);
I do understand how a Transform can "absorb" many transformation (Translate, rotate,scale...etc), and then merge the transform in a state or directly on a draw function.
But i have no clue what this matrix means?
I have been advised on another topic to use this matrix to do a rotation :
[cos(A) -sin(A)]
[sin(A) cos(A)]
I thought i could understand it later, but after rereading and trying to understand some SFML examples such the "tilemap" i still dont know what matrix have to do with Transformable.
2) ROTATION:
After re reading almost all turorials (except the "View" one) and trying to remember all graphics functions SFML have, i can finally say i dont know well how getRotation works.
Here an example of a rectangular shape with 2 other shapes i want to make them rotate AROUND the rectangle, the three shapes are named R (Rectangle), C(A triangle made with vertices) and O(and Object with a traingular shape, its the smallest and has one color, compared to the C triangle which is multi coloured).
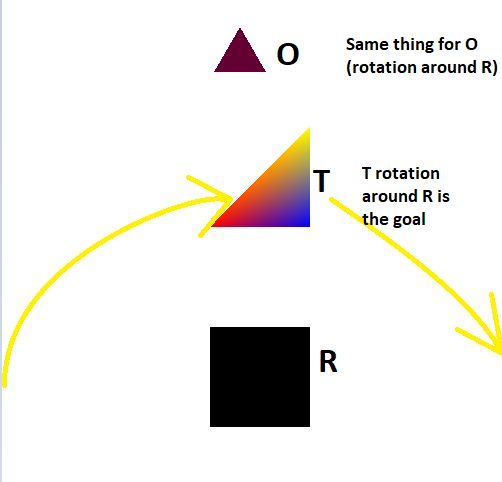
sf::RenderWindow window(sf::VideoMode(800, 600), "It Works!", sf::Style::Close, contextSettings);
window.setFramerateLimit(30);
///SHAPE1
sf::RectangleShape R; // rectangle
R.setPosition(450,350);
R.setSize(sf::Vector2f(100,100));
R.setFillColor(sf::Color::Black);
///SHAPE2
sf::VertexArray V(sf::Triangles,3); //Triangle closest to the rectangle
V[0].position = sf::Vector2f(R.getPosition().x,R.getPosition().y-100); // which is (450,350)
V[1].position = sf::Vector2f(R.getPosition().x+100,R.getPosition().y-100); // which is (550,350)
V[2].position = sf::Vector2f(R.getPosition().x+100,R.getPosition().y-200); // which is (550,250)
V[0].color = sf::Color::Red;
V[1].color = sf::Color::Blue;
V[2].color = sf::Color::Yellow;
///SHAPE3
sf::CircleShape O(30,3); // farest shape : object
O.setPosition(R.getPosition().x,R.getPosition().y-300);
O.setFillColor(sf::Color(100,0,50));
// newOctagon.setOutlineThickness(6.f);
// newOctagon.setOutlineColor(sf::Color::Red);
O.setOrigin(R.getOrigin());
O.rotate(45);
//R.rotate(45);
// triangle[0].color = sf::Color::Red;
while (window.isOpen())
{
sf::Event event;
while (window.pollEvent(event))
{
if (event.type == sf::Event::Closed)
window.close();
}
window.clear(sf::Color::White);
window.draw(R);
window.draw(V);
window.draw(O);
window.display();
So is it possible to make O which is a CircleShape, rotate around R?
Is it possible to make T which is a vertex type, rotate around R?
3)
Is it possible to fill multiple colours for regular shapes(not vertices) choosing areas with "Rect" ? (
Example like this :
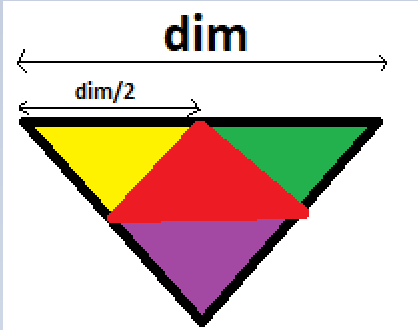
4) On the class sf::Unit8
I am not sure How it it used, because i find no classe called "Unit8" browsing the documentation: here an example i saw somewhere :
sf::Uint8* pixels = new sf::Uint8[width * height * 1]; // * 4 because pixels have 4 components (RGBA)
//sf::Uint8* pixels = new sf::Uint8[width /2];
// sf::Uint8* pixels2 = sf::Vector2f(400,0)...; // get a fresh chunk of pixels (the next frame of a movie, for example)
tex1.update(pixels); /// FIRST PROB
What happens to the Texture tex1 here?
5) A random vertices generator does nor work inside a new "MyEntity" class while drawing it on the window, but the code works outside the new class (inside main), this:
int N;
cout << endl << " choose N : (below 26000) " << endl;
cin >> N;
sf::VertexArray Vexy(sf::TriangleStrip,N);
srand(time(nullptr)); // use current time as seed for random generator
for(int i;i!=N;i++)
{
int random_variable2 = rand()%400+1;
int random_variable3 = rand()%500+300;
Vexy[i].position = sf::Vector2f(random_variable2+random_variable3, random_variable2/2);
Vexy[i].color = colorArray[rand()%5];
}
Result :
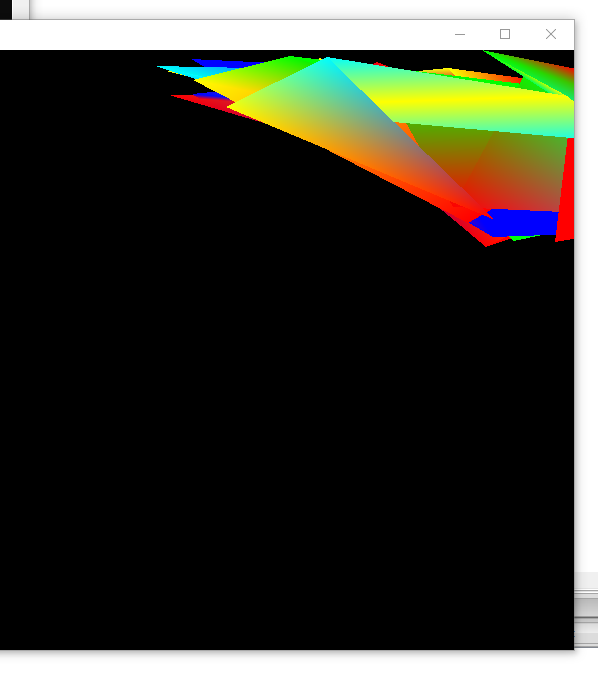
But while i m using a new classe, like this one, it does not work(does not show up with the function draw)
using namespace std;
class MyEntity : public sf::Drawable, public sf::Transformable
{
public:
MyEntity(float NewPointcount) : PC(NewPointcount)
{
sf::VertexArray Vex(sf::TriangleStrip, PC);
const sf::Color colorArray[5]={sf::Color::Cyan, sf::Color::Blue, sf::Color::Green, sf::Color::Red, sf::Color::Yellow };
///USING THE FUNCTION RAND => HASARD
srand(time(nullptr)); // use current time as seed for random generator
Vex[0].position = sf::Vector2f(0, 0);
Vex[0].color = colorArray[rand()%5];
for (int n=1; n != PC-1; ++n)
{
int random_variable = rand()%200+1;
int random_variable3 = rand()%600+300;
cout << "Random value on [0 " << RAND_MAX << "]: "
<< random_variable << '\n';
Vex[n].position = sf::Vector2f(random_variable, random_variable3);
cout << rand()%5 << endl;
Vex[n].color = colorArray[rand()%5];
}
Vex[PC-1].position = sf::Vector2f(0, 0);
Vex[PC-1].color = colorArray[rand()%5];
};
private:
virtual void draw(sf::RenderTarget& target, sf::RenderStates states) const
{
// apply the entity's transform -- combine it with the one that was passed by the caller
states.transform *= getTransform(); // getTransform() is defined by sf::Transformable
// apply the texture
states.texture = &m_texture;
// you may also override states.shader or states.blendMode if you want
// draw the vertex array
target.draw(m_vertices, states);
}
sf::VertexArray m_vertices;
sf::Texture m_texture;
int PC;
};
and inside the main :
MyEntity ME(6);
..
window.clear(sf::Color::Black);
window.draw(ME);
window.display();
Nothings shows up. Also the difference between using the CLASS and the exemple before is that i set the first and last vertices outside the "for".
6) My last question is about the "Tilemap" example :
Which can be found at the bottom of this page :
https://www.sfml-dev.org/tutorials/2.5/graphics-vertex-array.phpI had a hard time undesrtanding the the variables tu and tv, i think a sentence should be written as comment to describe them, it's a google search on them and made me fall on one of the old posts from SFML forum and made me understand that : "tu and tv are the tile coordinates of the texture. tu is the column of the tile, and tv its line."
Okay what does this line exactly do?
m_vertices.resize(width * height * 4);
I can read here :
https://www.sfml-dev.org/documentation/2.5.1/classsf_1_1VertexArray.phpthat the resize function purpose is to change the number of "points" or "vertices".
Dont we always need 4-5 vertices to draw a tile? Which is rectangular?
I really dont get this line i keep finding everywhere : "(width * height * 4)"
7) Also, i did an experiment, with adding a "cout" to know the location of the FIRST point of each tile + the location of the first TexCoord for each said point :
quad[0].position = sf::Vector2f(i * tileSize.x, j * tileSize.y);
cout<< " position 1 :" << i * tileSize.x << "-" << j * tileSize.y << endl;
quad[1].position = sf::Vector2f((i + 1) * tileSize.x, j * tileSize.y);
quad[2].position = sf::Vector2f((i + 1) * tileSize.x, (j + 1) * tileSize.y);
quad[3].position = sf::Vector2f(i * tileSize.x, (j + 1) * tileSize.y);
// define its 4 texture coordinates
quad[0].texCoords = sf::Vector2f(tu * tileSize.x, tv * tileSize.y);
cout<< " coordinates TEX 1 :" << tu * tileSize.x<< "-" << tv * tileSize.y << endl;
quad[1].texCoords = sf::Vector2f((tu + 1) * tileSize.x, tv * tileSize.y);
quad[2].texCoords = sf::Vector2f((tu + 1) * tileSize.x, (tv + 1) * tileSize.y);
quad[3].texCoords = sf::Vector2f(tu * tileSize.x, (tv + 1) * tileSize.y);
And i noticed this :
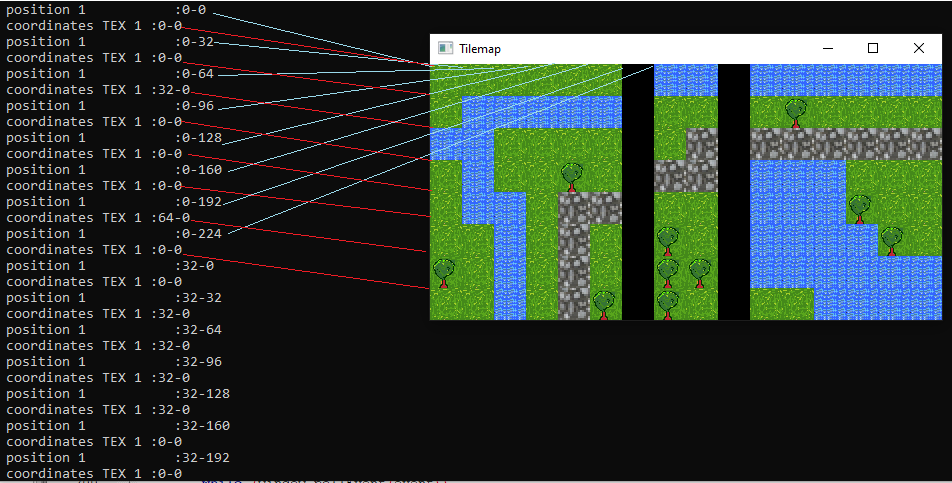
I still find it hard to understand it, i guess i will have to do many more examples to get it quicker, it seems that quad
.position thing browses line by line after finishing a line one after another (from left to right), while quad.texCoors seems to browse vertically (from up to down then it changes the collumn)
Anyway this last question was not really a question.