The position is changed with sf::Transformable::setPosition. The bounds are given by sf::VertexArray::getBounds(). How would these two things interact with each other without you doing something? How would you get a changing bounding rect magically without doing anything in your class?
sf::VertexArray::getBounds() simply returns the min/max of the vertices' coordinates. If you never change them, then the bounding rect will never change as well.
On the other hand, changing the position changes the translation components inside sf::Transformable, and in result, modifies the entity's transform (getTransform()).
Now... after correctly understanding all that stuff, how to write a getGlobalBounds() function that works similarly to SFML entities' getGlobalBounds()? Simply by transforming the vertex array bounds by the entity's transform. And taking the bounding rect of the result.
Very interesting! After reading your answer i tried "doing something" to changes the 4 variables defining the FloatRect obtained through getBounds(), so i did the following :
1) Store the FloatRect obtained with getbounds in 4 variables.
2) Each time the vertex moves, modify the first and second variables which were the initial "left" and "top" components of the floatRect. I never modify the "width" or "height" variables.
3) Use a FloatRect that contains the new "left" and "top" variables with the old width and height variables, and use it directly with "intesects".
So whenever my object moves, i get a FloatRect similar to the getGlobalBounds() i think? It seems to be working.
___ Now let's move to phase 2
Now to understand SFML better as you are suggesing me, maybe i should try to do it with "getTransform()) as you suggest.
To sum up, the transformable class (which my class derives from ) has the ability to use getTransform(), it generates an object from the class sf::Transform.
Its functions
https://www.sfml-dev.org/documentation/2.5.1/classsf_1_1Transform.phpSeems to have very few "getters" (but probably good enough), i don't see a getter that would give me information that can be applied to the vertexArrayObject.getBounds() to change it... I am sure there is but i don't see it.
It's probably inside the "getMatrix () const" function? which gives a matrix of four variables. I am supposed to use these 4 variables and apply them to the FloatRect obtained with GetBound(), then use the resulting FloatRect, i think?
Happax showed me a link to understand the 3x3 matrix and it's effects, i guess i have to dig more, but yeah i used another method and left the getTransform methode you are telling me about unfortunately,
i would like to be able to do the method you are describing.I really think you're going too fast. You're trying complicated stuff (it is!) without having a solid background (you thought it was caused by the "const" keyword, which doesn't make any sense).
Can't you work with SFML entities directly, until you understand SFML better? Why do you create your own entity class? What are you trying to achieve, what's your final goal?
True, since i understood how to inherit from the Transformable class i felt i can do anything.. and tried to do anything. Yes i am moving too fast, that's becuase i feel i am being too slow

.
So my goal (for now) is as follows : --- I have a big map containg.. let's say.. houses, like this :
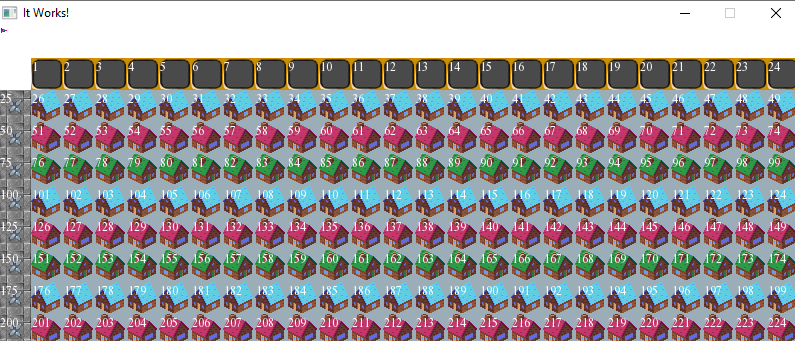
---Each house has a FloatRect.
---My vertixClass allows me to create any shape, the little object in the upper left is the shape (it's not exactly a triangle, it's a combinason of three VertixArray's).
I am using a vertexClass because i want it be able to expand the entity and make it smaller
at will, and i want it to have a specific shape, convex and concave. That's why i am not using the regular "shape" entities that SFML offers such as RectangularShape etc..
---What i want to do?
When my entity moves and reach a certain floatRect (which can be the green house or the red one or anything), something happens : a sound plays or the house is destroyed.
To test if the entity reached the house, you have to use the "intersects" function, and you have to obtain the entity bounds with getBounds (hence the beginning of this forum post).
So you can figure out the rest, i had a problem because getBounds did not give me a different FloatRect for each entity position, your solution seems to be interesting which is to play with getTransform() and which i am not sure how to use, in the meantime i tried to "save" all the "movements" the entity does, if the item goes "right" i can predict if it has reached a floatRect.. something like that. And it did the trick somehow. Not sure if it's the best way to do it?
Thank you for your answers and time, by the way. It's great to have this forum.