I'm not sure whether I do something wrong, or it's some undiscover SFML bug, but when I attach an additional texture to a shader bound to an image sprite it gets rendered normally, but when I do the same on a sprite with RenderTexture the additional texture bound to it appears flipped. Here is the link to the GitHub issue and the sourcecode I used.
https://github.com/SFML/SFML/issues/1733#include <SFML/Graphics.hpp>
int main()
{
sf::RenderWindow window;
window.create( sf::VideoMode::getDesktopMode(), "Flip bug", sf::Style::Fullscreen );
window.setVerticalSyncEnabled( true );
window.setMouseCursorVisible( false );
sf::Vector2u size = window.getSize();
sf::Texture texture;
texture.loadFromFile( "a.png" );
texture.setSmooth( true );
sf::Sprite texture_sprite;
texture_sprite.setTexture( texture );
texture_sprite.setScale( (float)size.x / texture.getSize().x, (float)size.y / texture.getSize().y / 2.0 );
sf::RenderTexture render;
render.create( size.x, size.y / 2 );
sf::Sprite render_sprite;
render_sprite.setTexture( render.getTexture() );
render_sprite.setPosition( 0, size.y / 2 );
sf::Shader shader;
shader.loadFromFile( "shader.frag", sf::Shader::Fragment );
shader.setUniform( "texture2", texture );
while (window.isOpen())
{
sf::Event event;
while ( window.pollEvent( event ))
{
if (( event.type == sf::Event::Closed )
|| ( event.key.code == sf::Keyboard::Escape ))
{
window.close();
}
}
render.clear();
render.draw( texture_sprite );
render.display();
window.clear();
window.draw( texture_sprite, &shader ); // this renders normally
window.draw( render_sprite, &shader ); // this renders flipped
window.display();
}
return 0;
}
shader.frag
// This shader draws texture on the left using RB channels and
// texture2 on the right using G channel
uniform sampler2D texture;
uniform sampler2D texture2;
void main()
{
vec4 color1 = texture2D( texture, gl_TexCoord[0].xy * vec2( 2.0, 1.0 ) + vec2( 0.0, 0.0 ));
vec4 color2 = texture2D( texture2, gl_TexCoord[0].xy * vec2( 2.0, 1.0 ) + vec2( -1.0, 0.0 ));
gl_FragColor = gl_Color * ( vec4(color2.x, color1.y, color2.z, 1.0 ));
}
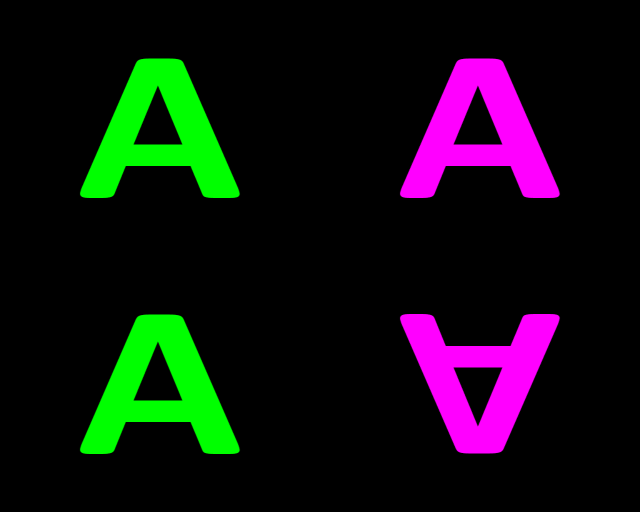