no need for a separate vector. also you don't need adresses if, for example, all sand tiles are going to be the same and do the same thing.
if you have a tilemap like:
tile_map = [[0, 0, 0, 1, 0,],
[0, 0, 0, 1, 0,],
[2, 2, 0, 1, 0,],
[2, 2, 1, 1, 0,],
[0, 0, 0, 1, 0,]];
and each tile is 32x32 pixels, all you have to do to get a particular tile ID is divide mouse position by tile size. after mouse clicking, it would be something like that:
//Inside your events loop
sf::Vector2i tile_size(32, 32);
if (event.type == sf::Event::MouseButtonReleased && event.mouseButton.button == sf::Mouse::Left){
int tile_id = tile_map[sf::Mouse::getPosition().x/tile_size.x][sf::Mouse::getPosition().y/tile_size.y];
}
EDIT: i was tempted to make a full working example

#include <iostream>
#include <SFML/Graphics.hpp>
int main(){
std::vector<int> tile_map = {0, 2, 3, 2, 1,
0, 2, 2, 2, 0,
0, 2, 0, 2, 0,
0, 0, 1, 1, 1,
0, 2, 1, 1, 0};
sf::Vector2i map_size(5, 5); //Number of rows and columns of the map. in this example must match the vector above, since I didn't add any checker methods to ensure everything fits well.
sf::Vector2i tile_size(32, 32); //Tile size in pixels
sf::Vector2i tex_tile_size(32, 32); //Original tile size in the texture file, in pixels
sf::VertexArray vertices(sf::Quads, map_size.x*map_size.y*4); //4 vertices for each tile
sf::Texture tileset;
tileset.loadFromFile("tileset.png");
sf::RenderWindow window(sf::VideoMode(map_size.x*tile_size.x, map_size.y*tile_size.y), "SFML Tilemap");
for (int y=0; y<map_size.y; y++){
for (int x=0; x<map_size.x; x++){
const int n = y*map_size.x+x;
sf::Vertex* quad = &vertices[n*4];
quad[0].position = sf::Vector2f(x*tile_size.x, y*tile_size.y);
quad[1].position = sf::Vector2f((x+1)*tile_size.x, y*tile_size.y);
quad[2].position = sf::Vector2f((x+1)*tile_size.x, (y+1)*tile_size.y);
quad[3].position = sf::Vector2f(x*tile_size.x, (y+1)*tile_size.y);
const int tile_id = tile_map[n];
const int tx = tile_id % (tileset.getSize().x / tex_tile_size.x);
const int ty = tile_id / (tileset.getSize().x / tex_tile_size.x);
quad[0].texCoords = sf::Vector2f(tx*tex_tile_size.x, ty*tex_tile_size.y);
quad[1].texCoords = sf::Vector2f((tx+1)*tex_tile_size.x, ty*tex_tile_size.y);
quad[2].texCoords = sf::Vector2f((tx+1)*tex_tile_size.x, (ty+1)*tex_tile_size.y);
quad[3].texCoords = sf::Vector2f(tx*tex_tile_size.x, (ty+1)*tex_tile_size.y);
}
}
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
if (event.type == sf::Event::Closed){
window.close();
}
if (event.type == sf::Event::MouseButtonReleased && event.mouseButton.button == sf::Mouse::Left){
sf::Vector2i current_tile(sf::Mouse::getPosition(window).x/tile_size.x, sf::Mouse::getPosition(window).y/tile_size.y);
int tile_id = tile_map[current_tile.y*map_size.x+current_tile.x];
std::cout << "You clicked tile [" << current_tile.x << "][" << current_tile.y << "]. ID of this tile is " << tile_id << std::endl;
}
}
window.clear(sf::Color::White);
window.draw(vertices, &tileset);
window.display();
sf::sleep(sf::milliseconds(10));
}
return 0;
}
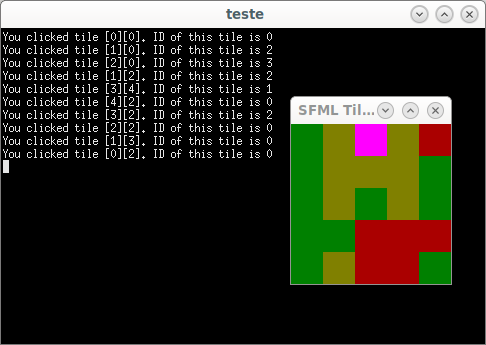
have fun!