I am trying to recreate pong to help me along with my learning on C++ and SFML, but I've ran into a few problems when trying to make the ball move and bounce.
I managed to get the ball to move on its own, and to bounce off the walls and the paddles easily, but the problem was that it didn't seem "realistic". The ball was always bouncing at the same angle, as what I did was this:
float velX = 0.15f;
float velY = 0.35f;
// Move the ball in the game loop
Ball.Move(velX * App.GetFrameTime(), velY * App.GetFrameTime());
// When the ball hits the top or bottom of the screen
velY = -velY;
// When the ball hits a paddle
velX = -velX;
Doing it like this meant that the user has no control over where the ball goes, as it would just bounce around at the same angle and be pretty boring.
I reasoned that to do this I'd need to use trigonometry, so I attempted that.
Correct me if I'm wrong, but this is what I did to try and work it out:
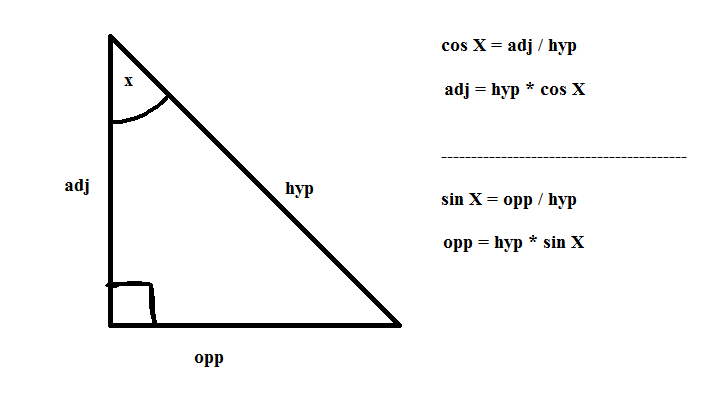
where x is the angle I want the ball to move, and hyp is the ball's speed?
So to move the ball I would do:
Ball.Move(App.GetFrameTime() * ballSpeed * cos(ballAngle), App.GetFrameTime() * ballSpeed * sin(ballAngle));
Is this correct? And excuse the cos and sin bits there, I don't know how to do those in C++ yet.