That's a really good tutorial, I didn't know views have so many usages, and I've learnt a couple of things about viewports (that's a new feature, right?).
Until now, for split screens, I used a very different approach: a parent container inheriting sf::Transformable & sf::Drawable, and I apply the container's transformations on its children, in the overridden draw method.
class Container: public sf::Transformable, public sf::Drawable
{
void draw(sf::RenderTarget& target, sf::RenderStates states) const
{
states.transform *= getTransform();
for each child
{
target.draw(child, states);
}
}
};
Should I use views instead? Is one method faster than the other?
Also, why the viewport rectangle is defined using ratio instead of dimensions in pixels? This puzzles me.
For example, if I want to split my screen between a game zone and an HUD zone like this:
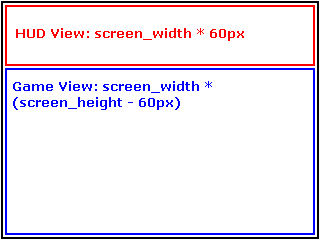
This leads to the following:
float h = (screen_height - 60) / screen_height;
sf::View game_view(sf::FloatRect(0, 0, screen_width, screen_height - 60));
player_view.setViewport(sf::FloatRect(0, 1 - h, 1, h));
sf::View hud_view(sf::FloatRect(0, 0, screen_width, 60));
hud_view.setViewport(sf::FloatRect(0, 0, 1, 1 - h));
So the ratio values are rounded, and I'm afraid this may result in non-pixel perfect positioning.