I have this in a language file encoded in UTF-8:
<common
main_menu_start="Begin"
main_menu_arcade="Arcade"
main_menu_options="Options"
main_menu_about="About"
main_menu_quit="Quit"
about_made_by="A game by:"
about_rasmus="Rasmus Riiner - Programming and the rest"
about_mona="Mona Liinat - Background Images"
about_oliver="Oliver Kütt - Music and Support"
loading="Loading..." />
These are the relevant bits in my code:
const sf::String Game::GetText(const char *category, const char *line) {
TiXmlElement *Category = langFile.FirstChildElement(category);
sf::String string = Category->Attribute(line);
return string;
}
/.../
aboutOliver.setString(Game::GetText("common", "about_oliver"));
The outcome of this code:
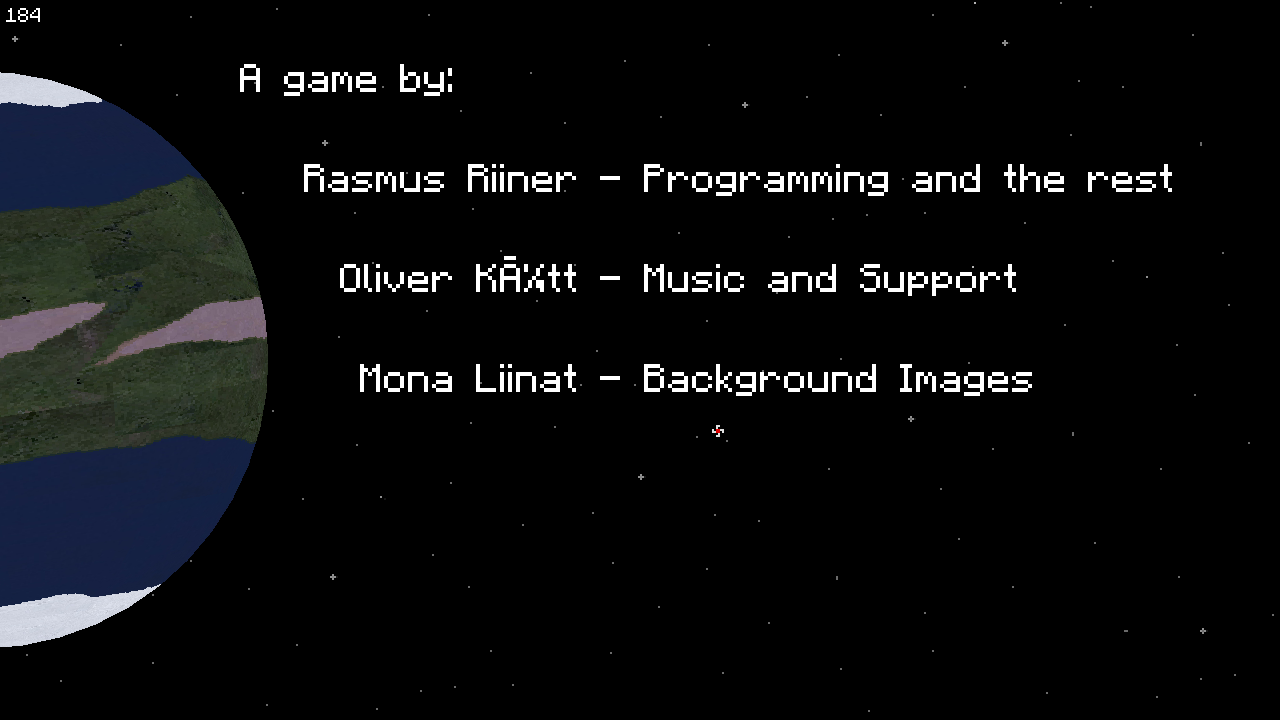
So, how would I fix this problem of mine? :]
Thanks in advance.