I'm making an adventure game where you type in text commands in English, like this:
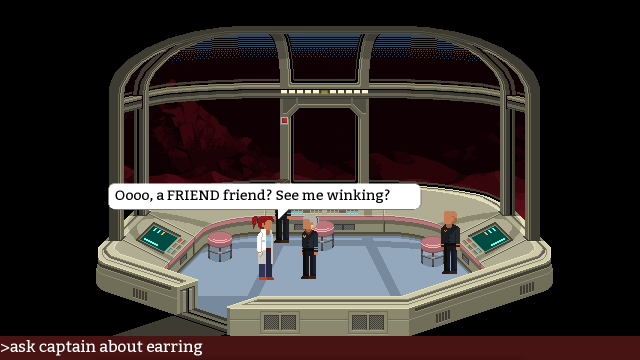
I know this is broad, but are there any specific practices or considerations to handle non-English keyboard layouts in my code?
My command input only accepts a-z, 0-9, space, and backspace, so per the SFML tutorial my game loop looks like this:
if (event.type == sf::Event::TextEntered) {
if (event.text.unicode < 128) {
char charEntered = static_cast<char>(event.text.unicode);
if ((charEntered >= 'A' && charEntered <= 'Z')
|| (charEntered >= 'a' && charEntered <= 'z')
|| (charEntered >= '0' && charEntered <= '9')
|| charEntered == ' '
|| charEntered == '\b') {
// Show the next letter...
}
}
}
In other words, I toss out everything except a handful of ASCII chars.
Does anyone see any pitfalls in this approach with respect to foreign keyboards? Do you think this is generally sufficient so that someone using a French layout, or a bilingual Hindi/English keyboard, will be able to type in English correctly?
I'm not well versed in internationalization issues, so any non-English insight is appreciated.