Okay, I finally got around to releasing this thing.
This library provides scalable truetype font support for SFML.
sf::String does this but in a different way, and does not support a few of the features this library provides (yet!).
Right now this has only been tested with the newest SVN!
I'm not sure if it would compile/work with older versions.
This is intended as an alternative to SFML's sf::String until SFML supports outlines, etc.
Screenshots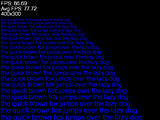
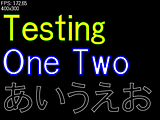
Features-Kerning support
-Outlined/stroked text
-Sizeable outlines
-Unicode
DownloadI actually set up a Google Code project page for it since it seemed a little large for a wiki (and I wanted SVN).
http://code.google.com/p/sfttf/downloads/listsfttf-0.2 comes with precompiled libs/test program.
Example
sfttf::FontManager FontMgr;
FontMgr.Initialize();
sfttf::Font* Font = FontMgr.loadFont("Font.ttf");
Font->setOutline(true);
Font->setFill(false);
Font->drawString("Hello, World!", 300.0f, 300.0f);
Everything is in the sfttf namespace.
You need a FontManager to load fonts (FontManager::loadFont).
The FontManager will unload all the fonts upon destruction or you can call FontManager::unloadFont.
See the readme/example for more info.
Project files are provided for Windows+Linux (codeblocks/vc2008).
NotesRight now there are a few simple things that I have not added like rotations.
It only supports horizontal text, it does not support vertical layout.
It probably does not work with languages like Thai that have multiple levels per line.
It does not use exceptions. If something can fail, a boolean is returned.
I removed support for control characters '\r\n\t\v' (etc) at the last second as it complicated a few things.