Alright, I'm stumped. It's been three days and I'm running out of options - I'm to the point of just trying random, seemingly pointless stuff just to get something to work.
I'm writing a program that binds to Lua and allows scripts to draw things to the canvas. The code is completely custom and
is too large to simplify down (sorry Laurent, this one won't be easy).
I have tried running a bare-bones text drawing program, which, of course, works.
I have a font preloader (which does exactly what it sounds like - creates a font and loads from a file, stores it in an array, and then returns a
raw pointer [Lua uses raw pointers]), as well as an image loader that works pretty much in the same way.
Images have a wrapper class, whereas the sf::Font class is bound directly to lua (no wrapper).
There is also a text class that lightly wraps the sf::Text class.
Text was working intermittently (certain, seemingly similar builds would show/hide text at random) until it just stopped working altogether.
The text just seems to be completely invisible.
I have stepped through the program to the point my F10/11 keys are screaming for mercy, and all pointers/references/arguments/etc. seem fine. I have compared runtime break data for Fonts between the working sample program and the actual app - everything looks fine. There are absolutely no errors or exceptions, the text simply does not display.
The other weird thing is that
images draw just fine. They also follow the same code-path as text does. It seems to be an internal thing.
Here is a few code snippets to help visualize the code-path:
Button (which is what holds the text) instantiates the label (wrapper Text class):
Text _label;
which instantiates itself using the sf::Text default constructor. The button class then sets the label's string and calls the lua script to set itself up.
_label.setString(std::string("Test"));
-- Get Label
local label = self:GetLabel()
-- Set up font/color
label:SetColor(R.Color(255, 0, 0, 255))
-- _buttonFont is a font that was pre-loaded earlier in the script
self:GetLabel():SetFont(_buttonFont)
The above lua code works as far as I can tell; Even deeper into the draw functions, the color values are correct (255,0,0,255) and the font seems to be normal (the pointers are valid, the page count is 1 or so, and the pixel data is reasonable).
The code then continues on to draw, calling the lua callback with a reference to the target (which wraps a pointer to a rendertarget):
-- Draw image then text on top of it
-- Commented out image drawing; It works fine
--target:Draw(_buttonIdle)
target:Draw(self:GetLabel())
The target draw method is as follows:
ITarget& draw(Drawable& drawable)
{
// Is it visible?
if(!drawable.isVisible())
return *this;
// Init
initStack();
// Combine transform
_transformStack->push(_transformStack->top());
_transformStack->top().combine(drawable.getTransform());
// Draw
drawable.drawThis(*this);
// Pop Transform
_transformStack->pop();
// Return
return *this;
}
It is confirmed the drawable is visible (which is a method that belongs to a wrapper) and the transform stack (used to combine transforms for 'component'-ized drawables) is initialized and working fine.
The code goes on to draw the drawable's wrapper function, which calls the pure virtual method "onDraw" that actually does the drawing.
// Draw
target.drawRaw(*this);
drawRaw() simply calls another target wrapper draw method to call the SFML draw method itself:
// Copy + Combine states with transform
sf::RenderStates s = states;
s.transform.combine(_transformStack->top());
// Lock and load
lock();
_ref->draw(drawable, s);
unlock();
Again it pushes the transforms, etc. and locks (thread and GL states, including setting the target to be active) and draws.
This triggers the SFML sf::Text draw method. The m_font information at that point looks like this:
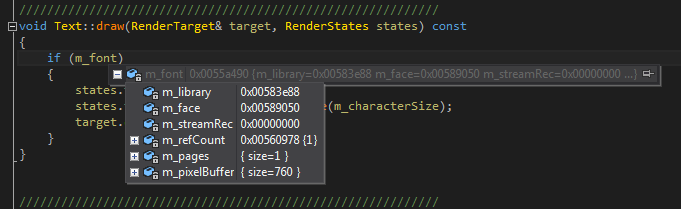
Beyond that, everything seems fine. Like I said, the color is there, the font information looks fine, and with the help of notepad+watch lists, I've confirmed all pointers are as they should be.
I seriously don't know why text is just invisible.
---
My question isn't 'how do I fix this' or 'what's wrong with my code' (since condensing this to something simpler would be pointless) - but more of
how can I begin to debug text drawing? I have already updated GLEW and Freetype, building from source, and I'm using the latest (as of a few hours ago) dev snapshot of SFML 2.0.