Recently Updated! (AGAIN) WOOHOO V0.0.80 is now released!!Edit: Wiki now added for recipes!http://xsgteam.net/ParadoxWiki.phpWhat is Paradox?Paradox is a game based on a Zombie Apocalypse.
It is a top-down 2D shooter, with an RPG aspect.
Currently, you have one goal...Survive.
You can find Metal/Wood, and craft guns to stay alive, but you also have to craft ammo!
There is Blood, Fog/Clouds, Zombies, and Day/Night system with a Day counter.
This game is in PRE-Alpha, meaning it's no where near close to being finished, and will continue to get better!
You can craft via putting items in the crafting box in a certain pattern or "recipe". We give you 2 right away in the read_me file, so check it out.
screen shots:
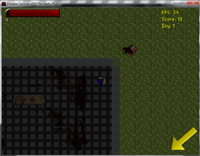
Default Controls
---------------------------------
Left mouse click -- Shoot / Place Wall (or other objects)
Right mouse click -- Punch / Activate Work Bench / Open Doors
Shift -- Run/Sprint (Gains momentum overtime)
W, A, S, D -- Movement Controls (Changable)
I -- Inventory (Unchangable)
M -- Rotate Object (Placeable Object) (If in HotBar and Selected)
O -- Opens up debug information.
R -- Manual Reload (Changable / Uses current ammo)
Escape -- Exits out of any menu/game state.
How to gather resources?
---------------------------------
Move on top of an resource and press Space once.
A progression bar will be displayed over head.
Once progression bar is filled (100%), a random resource will
fall on to the ground (which you can pick up and use)
Thanks for checking it out.
To login to game, you'll have to make an account on our site, but it IS free

(Offline mode is there though

)
Small list of Updates from 0.0.60 - 0.0.80 (Can't fit all

)
-NEW! Zombie AI! (Zombies now are smarter then eever!)
-Did much optimization with views (More fps)
-New appeal to Inventory/Crafting Slots and Screens
-Added Design for Main Menu/Login Screen (more features as well)
-Added Modding system added (For weapons, which increases certain stats of a weapon)
-Added Shop system (Enables you to sell resources and gain money to buy items! (mods/weapons/rares)
-Added Currency system (for shop system!)
-New Collision system for everything (Easier to get by smaller places! And less glitches!)
-Added building system (allows you to build with blocks you either make or buy)
-More work done with profiles (Longest day survived/Most money/Total score/Total kills/etc!) (only for users logged in)
-House generation (with random houses generated with goodies inside the house!)
-More items to craft (Think floors/Walls/minor-mods/weapons/etc)
-Hunger system! (Food heals you and it can kill you if you don't eat!)
-More information sent from the game to your profile at our site! (stats!) (
http://xsgteam.net)
-Fixed a bunch of bugs when you could view things in Pause/Death screen!
-Also new variable handles which gui box displays first! (So hover over health and crafting box, wont display both)
-Added sfx (when buying/selling items)
-Added sfx (when punching zombie!)
-Added sfx (when drinking drink)
-Added sfx (when eating burger)
-Added option in menu to turn off tips.
-Made some new sounds (un-used atm, for cellslots)
-Made weapons sell value depend on durability!
-Some new hints/tips added!
-Tips/hints regenerate randomly every 30 seconds
-Successfully added menu (in-game)
-FIX: In Store the weapon will display sell value (in your inventory now)
-FIX: Bunch of bugs with zombiess!
-FIX: Moved ammo to Health Bar
-FIX: If Intersection with HotBar you will not be able to shoot!
-Removed Day/Night Orb because of problems! Will work on more...
-Added a lot more! (Check ittt ooout)
New Updates from 0.0.53 to 0.0.60
-Remade crafting system!
-Fixed up some GFX
-Durability added for weapons!
-Certain key items do not stack anymore! (Weapons, etc)
-Zombie AI made (Zombies now can chase you after sight!)
-NO zombies get spawned day one! (Go gather resources, fool!)
-Console added, for when logged in (Press T!)
-Fullscreen mode added! (Not for main-menu though) (FPS may drop depending on computer)
-Splash screen
-Save / Load fixing / tweaking (Current saves will not work

)
-Added zombie Brains (Zombie bait!) (1/20 chance dropped when kill)
New Updates from 0.0.51 to 0.0.53
Here's the changes:
-Alerted when picked up an item.
-New save/load system!
-Better Item System
-Resources now can be found in multiples!
-More solid engine
-GFX modifications for Inventory
-GFX updates in general! (icon fixes, etc)
-Fixed rifle recipe (and Winchester)
-More debugging stuffs!
New Updates from 0.0.50 to 0.0.51:
-Gathering stopped when shooting/punching
-Added Scrap Rubber!
-Added more recipes. (Changed recipes to require rubber)
-Added more gui to crafting! (And crafting button!)
-Added rifle (allows rifle/winchester!)
-Added night/day gui (orb)
-Fixed crafting bugs
-Fixed collision bugs
-Offline mode
Check out more details here:
http://xsgteam.net/Download:
http://xsgteam.net/Paradox/Paradoxv0.0.80P.zipBIG thanks to Laurent for making an awesome lib (SFML)!
----------------Notes---------------
Current saves from past versions will not work, due to editing of saving/loading.
Do not run Updater Manually, unless you have a bad version and you need to redownload
all old files. Thanks.
Please Note, this game is in Pre-Alpha mode and may contain
bugs and glitches. So please be aware, and you are playing this game
at your own risk. Report all bugs/glitches to us (
http://xsgteam.net/ParadoxBugs/)
OR direct e-mail to (brett@xsgteam.net or james@xsgteam.net)
When sending bug report, please press "o" in game to bring up debug-display.
Then take a screenshot, and send to us,
Along with instructions on how to recreate bug. Thanks.