The post is a bit long, if you don't want to read it all, voting on the polls at the bottom of my
home page will already help me a lot. But I hope that some people will take the time to read it and give me their opinion about how the new callback system should be implemented.
First a small explanation on how the new callback system will work.
The current implementation is just polling the callbacks from the window, which is only a small part of the new system.
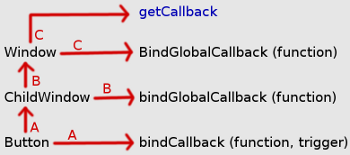
When a callback is triggered (e.g. the button has been clicked) then the following process will happen:
A: If bindCallback was used to bind a function to the callback trigger (mouse click), then the function will be called.
When a callback was requested for the trigger which isn't bound to a function (a bindCallback call were only the trigger was passed) then the callback will be passed to the parent object.
B: If a global callback function was set then this function will be called. Otherwise the callback will again be passed to the parent until it reaches the window.
C: If a global callback function was set then this function will be called. Otherwise the callback will be added to the callback queue and you will later be able to poll it from the window with getCallback.
When starting implementing it, I found out that it's not a big problem to bind multiple functions to one trigger so forget what I said before about binding only one function. You will be able to bind as many functions as you want to.
There are still a few things that could be changed.
First of all, I am not sure if bindCallback is a good name. Functions aren't really bound as you can connect multiple functions and calling the function with only the trigger parameter won't bind anything, it just tells the object to warn its parent on a callback.
Secondly, wouldn't the name pollCallback be better than getCallback (looks more like pollEvent)?
I have added polls for this on my site, so you can tell what you think is best there.
That leaves one last issue. When either in A, B or C, the callback is send to a function, it will never reach the callback queue. To illustrate it in the picture: if an arrow to the right is followed, then the process will stop and not continue going up.
This might be what you want, but in some situations you might want to silently bind a function, so that you are alerted about the callback but the process still continues.
I could make callback functions return a bool to signal if the callback has been handled or if it should continue to the other callback functions. An other option would be always go through all steps and don't stop when one callback function is called, but this means that all callback will reach the queue in the window and you must thus always poll the callbacks, even if you already handled them in different functions.
Any opinions on what the best option would be?